Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial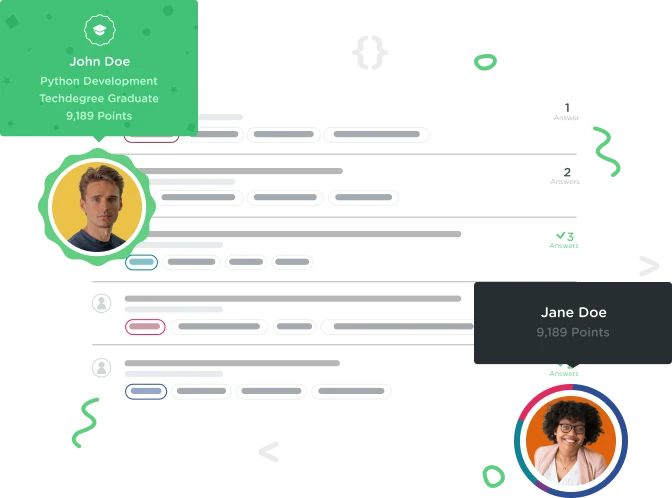

CRIS ACOSTA
2,121 PointsWhy is the if - else statement not working on this challenge?
So every time I search a student's name regardless if the student's name exist within my object array it always brings up the 'student not found' alert box. I am using if else statement.
Please help understand why this code is not working.
var search;
var studentReport = '';
var student;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function studentRecord(obj){
var report = '<h4>Name: ' + obj.name + '</h4>';
report += '<p>Track:'+ obj.track + '</p>';
report += '<p>Achievements'+ obj.achievements + '</p>';
report += '<p>Points:'+ obj.points + '</p>';
return report;
}
//'while' loop allow the program to continually ask to
//enter student name until user types quit
while(true){
search = prompt('Enter student name i.e "Dave" or type "Quit" to quit.');
if(search === null || search.toLowerCase() === 'quit') break;
for (i=0; i<students.length; i++){
student = students[i];
if(search.toLowerCase() === student.name.toLowerCase()){
studentReport += studentRecord(student);
print(studentReport);
}else alert('student not found');
break;
}
}
4 Answers
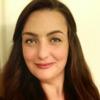
Jennifer Nordell
Treehouse TeacherHi there! In your for
loop, you are looking at each student to see if the name matches the name being searched for. Let's suppose you're searching for "Ben", and your first student is named "Ben". Great! It runs the print function with "Ben's" student report. Then it moves on to the next student. Let's say student #2 is named "Anne". Anne is not equal to Ben and the alert shows and the loop exits.
To fix this, you would need to display that no students have been found after you've checked the entire list and no result was found. The way you have it now, the only way it would not show the alert is if the list did not contain a student who did not have the name being searched for.
Hope this helps!

CRIS ACOSTA
2,121 PointsThanks Jennifer Nordell !
Here is the finished code
var search;
var studentReport = '';
var student;
var found;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentRecord(obj){
var report = '<h4>Name: ' + obj.name + '</h4>';
report += '<p>Track: '+ obj.track + '</p>';
report += '<p>Achievements: '+ obj.achievements + '</p>';
report += '<p>Points: '+ obj.points + '</p>';
return report;
}
//'while' loop allows the program to continually ask user to
//enter student name until user types quit
while(true){
search = prompt('Enter student name i.e "Dave" or type "Quit" to quit.');
found = false;//assume student value entered is not found
if(search === null || search.toLowerCase() === 'quit') break;
for (i=0; i<students.length; i++){
student = students[i];
if(search.toLowerCase() === student.name.toLowerCase()){
studentReport += getStudentRecord(student);// '+=' appends 'student' value to 'studentReport' instead of just assigning it which means that each student name searched will show when 'print' function is called.
/*using '=' only assigns value to studentReport which means that it will overide the last student value and will only show last matched value when print function is called.*/
print(studentReport);
found = true;
}
}
//if value is false alert user student is not found.
if(!found){
alert('Student not found!');
}
}
Also credits to:
I can finally move on to the next challenge after a week of this. I guess after this course I will run out of hair to pull. lol.

Heidi Puk Hermann
33,366 Pointsfrom your code, it appears that you are missing your students array... In that case your conditional will always return false.
just disregard :)
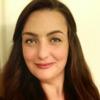
Jennifer Nordell
Treehouse TeacherHeidi Puk Hermann the students array is found in a separate JavaScript file which is linked in the HTML. Had it not been able to read the students
array the student who posted the question would have received an error about students
being undefined.

Thomas Grimes
10,189 PointsThank you everyone. I was trying the exact same approach as Cris and this was really hanging me up. So by setting the var found value to 'true' after finding a student, that's how we're able to skip the alert?