Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial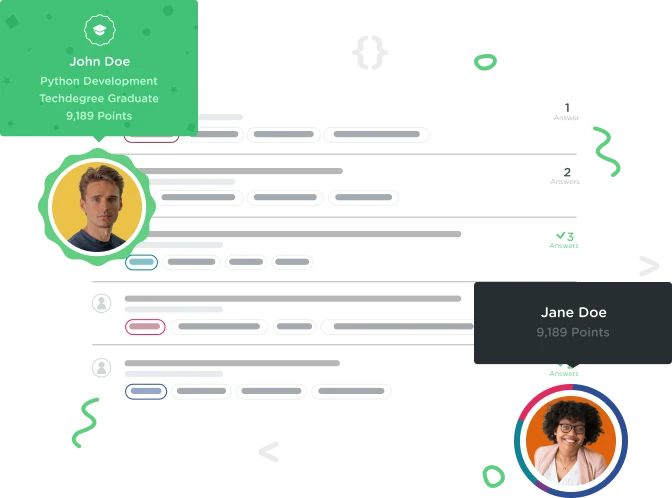

William Rodriguez
1,923 PointsWhy is the "let" necessary in the if-let statement? Wouldn't if just work fine without it? Why make it a constant?
If you could explain it in the context of the if let culprit = ... context, that would be great.
1 Answer
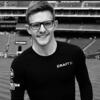
agreatdaytocode
24,757 PointsHello William,
When to use “if let”?
If let is a special structure in Swift that allows you to check if an Optional holds a value, and in case it does – do something with the unwrapped value. Let’s have a look:
if let nav = controller.navigationController {
nav.pushViewController(myViewController, animated: true)
} else {
//show an alert ot something else
}
The if let structure unwraps controller.navigationController (i.e. checks if there’s a value stored and takes that value) and stores its value in the nav constant. You can use nav inside the first branch of the if. Notice that inside the if you don’t need to use ? or ! anymore. It’s important to realize that nav is actually of type UINavigationController that’s not an Optional type so you can use its value directly. (If you remember in contrast the navigationController’s original type is UINavigationController?)
if let is useful also in another case. Imagine that instead of a single method call you want to do more stuff with your navigationController. If you do not use if let you code will looke like this:
controller.navigationController?.hidesBarsOnSwipe = true
controller.navigationController?.hidesBarsOnTap = true
controller.navigationController?.navigationBarHidden = false
controller.navigationController?.popToRootViewControllerAnimated(true)
This code works but you need to unwrap the property 4 times and the code gets quite repetitive. It’s much cleaner and nicer (and faster) to unwrap the value once in an if let:
if let nav = controller.navigationController {
nav.hidesBarsOnSwipe = true
nav.hidesBarsOnTap = true
nav.navigationBarHidden = false
nav.popToRootViewControllerAnimated(true)
}
Much nicer, isn’t it?
If you liked to learn more check out this post.
HaeJu Lee
3,811 PointsHaeJu Lee
3,811 PointsI have a question here, and I am new to swift.
on the 3rd snippet, there are '''swift if let nav = controller.navigationController { nav.hidesBarsOnSwipe = true } '''
I am somewhat curious and confused with the concept of pointers in other programming languages.
at let nav = controller.navigationController, is nav just another copy of controller.navigationController, or controller.navigationController itself(sort of referenced)?
so when nav.hidesBarsOnSwipe = true read,
controller.navigationController.hidesBarsOnSwipe actually turn to true? or just nav.hidesBarsOnSwipe turn ture?