Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial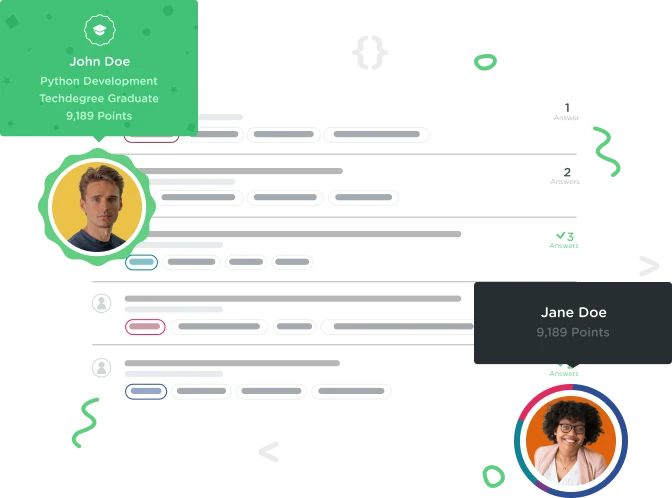

Forrest Pollard
1,988 PointsWhy is the OR operator not working in the way I used it
Hello!
My code is using the OR operator covered in a previous lesson. The code is to detect if either input is NaN. If one of them is, the code runs anyway however if BOTH are NaN it brings up the alert like i want it to and I get the intended outcome. Why is this happening?
Here is my code for your inspection
let numGen;
let numGen2;
let placeHold;
let rNum = prompt("choose first number"); //floor number
rNum = parseFloat( rNum );
let rNum2 = prompt("choose number 2"); //ceiling number
rNum2 = parseFloat( rNum2 );
if ( rNum > rNum2) {
alert("error, input 1 exceeds input 2");
} else {
placeHold = rNum2 - rNum;
if ( isNaN(rNum || rNum2 || placeHold)) {
alert("error, input is not numeric");
} else {
numGen = Math.floor( Math.random() * placeHold ) + 1;
numGen2 = numGen + rNum;
document.write(`${numGen2} is random number 'tween ${rNum} and ${rNum2}`);
}}
thanks in advance :)
1 Answer
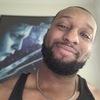
Brandon White
Full Stack JavaScript Techdegree Graduate 34,660 PointsHi Forrest,
In this case the OR (||) operator is not doing what you're intending it to do. Not sure how far a long in your studies you are, but it might make sense to explain it to you this way.
Say you were declaring and initializing a variable. Let's name the variable foo.
let foo = 6 || 'cake' || True
console.log(foo)
// output: 6
The reason the output is 6 is because 6 is Truthy.
Here's a different example.
let bar = 0 || 'animal' || 'brown'
console.log(bar)
// output: 'animal'
The reason the output is 'animal' is because 0 is Falsy, but 'animal' is Truthy.
In both of those cases, the OR operator makes it so that the first Truthy value is stored as the value that the foo and bar variables hold.
So when you write isNan(rNum || rNum2 || placeHold)
this code isn't actually checking if any of the three values are Not a Number, it's only checking if the first Truthy value is Not a Number. So if rNum is a string, the the result is True (because all strings are Truthy, the other two values (rNum2 and placeHold) aren't even checked), and then the string (which is what rNum is in this pretend example) is evaluated to determine if it is Not a Number. It is true that a string is Not a Number, and so the alert would be called.
That scenario probably works the way you want it to, but here's the rub. Say rNum is 6, but rNum2 is a string. Well rNum is Truthy (because any number that's not 0 is Truthy), so 6 is what's passed into the isNaN() function. The javascript interpreter never even processes the rNum2 variable to learn that it's a string. And since 6 is a number, the test returns false, and your alert is never called.
To make your code work the way you're intending you'll have to use three different isNaN functions (i.e. isNaN(rNum) || isNaN(rNum2) || isNaN(placeHold)
). Does that make sense?
TLDR;
Essentially using the OR operator inside the parens of the function is only determining which of the three values will be passed in as an argument to the isNaN function. It isn't checking all three to verify that they are all numbers.
Forrest Pollard
1,988 PointsForrest Pollard
1,988 Pointsdude thanks so much. I didn't expect to get a best answer straight away but that was unbelievably helpful. Thank you for not only telling me why it didn't work but also helping me fix it too.
P.S Also I agree that my original code was "fubar" hahaha