Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial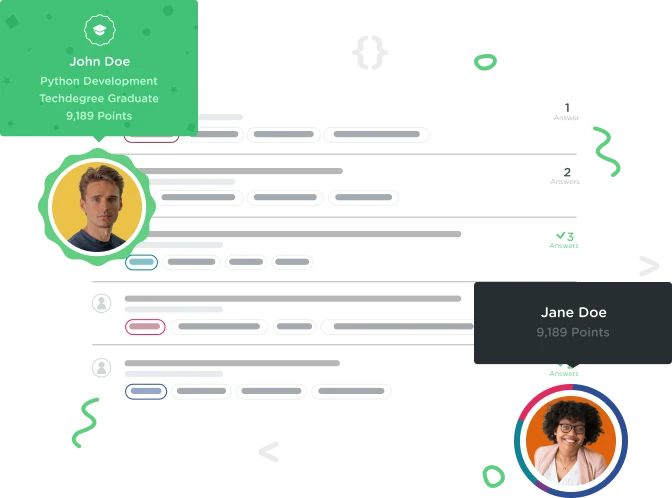
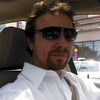
Ron L
6,463 PointsWhy is the print "Enter your name..." and name = gets.chomp in the loop? They're not needed for looping.
I pulled the print and gets.chomp out and it works fine. They aren't really necessary in looping, unless I'm missing something obvious.
def get_name
print "Enter your name (minimum 2 characters, no numbers): "
name = gets.chomp
loop do
if name.length >= 2 && !name.index(/\d/)
break
else
puts "Name must be longer than 2 characters and not contain numbers."
end
end
return name
end
name = get_name()
puts "Hi #{name}."
1 Answer

Freddy Heppell
9,753 PointsThe reason for this is so that it continually prompts you for your name until it meets the requirement of more than two characters.
Try entering a name of less than two characters, what happens?
- You enter the name "a"
- The loop starts
- The name is less than two characters, so it prints the warning
- The loop runs again
- The name is still less than two characters, so it prints the warning
- The loop runs again
- The name is still less than two characters, so it prints the warning
This will repeat forever, you have created an infinite loop.
The reason that the name request is in the loop is so, if the name is formatted incorrectly, it can ask you again, and repeatedly ask you until you have entered a valid username. Hope this helps.
JoJo KoKo
4,712 PointsJoJo KoKo
4,712 PointsPrint and setting the variable name is not part of the loop. It places the data that you received into the variable name and does loop around the variable name.
The loop part of this method checks if character is less than 2 characters and returns if greater than 2 characters.