Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial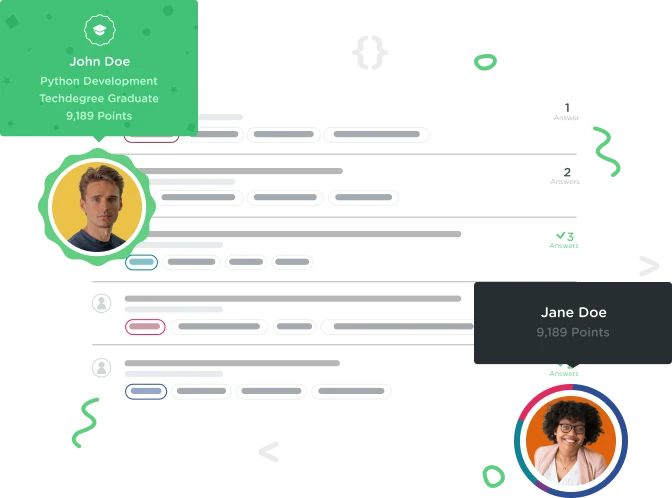

Britton Zirkle
Courses Plus Student 15,005 PointsWhy is the Prototype necessary?
What is the purpose of using the prototype when you can use constructor notation to define the properties and methods you wish to use in instances of the object? for example
var personPrototype = {
name: 'Anonymous',
greet: function (name, mood) {
name = name || "You";
mood = mood || "good";
console.log("Hello, " + name + " I am " + this.name + " and I am in
a " + mood + " mood!");
},
species: 'Homo Sapien'
};
function Person (name) {
this.name = name;
}
Person.prototype = personPrototype;
jim = new Person("Jim");
as opposed to
function Person (name) {
this.name = name;
this.species = 'Homo Sapien';
this.greet = function (name, mood) {
name = name || "You";
mood = mood || "good";
console.log("Hello, " + name + " I am " + this.name + " and I am in
a " + mood + " mood!");
};
}
var jim = new Person('Jim');
Unless I am missing something, using the constructor notation seems to save a lot of code and time.
2 Answers
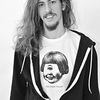
eck
43,038 PointsYou're right, you can declare your properties and methods in the constructor, and your code will work as expected. From my understanding though, you have small performance improvements when using the prototype to store common data and methods.
Check this stack overflow thread that basically answers the same question you have asked.

Olga Filatova
8,045 PointsNot only it's good for performance and cascading changes but also when using prototypes you can set one prototype to different classes or prototypes.
For example here we can declare prototypes like "american", "briton", "ukrainian", etc. linking to prototype "person" and for each inheriting prototype declare their own methods and properties. And so on, and so on.
Imagine which code will become larger in such circumstances.
In JS for example String, Number, Array, etc. prototypes inherit from Object prototype.
And winning on performance is not little. Especially in big JS projects. In having link to prototype in object or whole bunch of repeating code inserted in every little variable is a big differnence. DRY. Have mercy on RAM and your user. :)
Britton Zirkle
Courses Plus Student 15,005 PointsBritton Zirkle
Courses Plus Student 15,005 PointsErik,
Thanks for the info. You are correct. I also found out that using prototype allows it to act more like a class in which a change in the prototype will cascade to all objects created from that prototype which is pretty cool.