Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial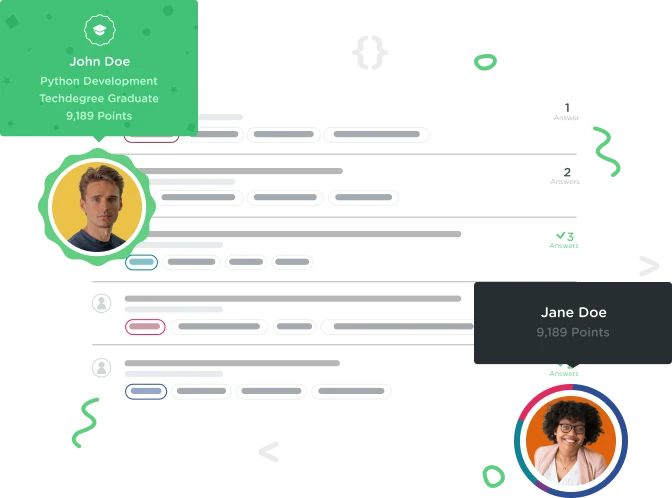
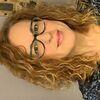
Rachael Zordan
1,379 PointsWhy is the return keyword not used if omitting curly braces in arrow functions, but it IS used if keeping curly braces?
I have completed creating random number function challenge using Function Declaration, Function Expression, and a few variations on Arrow Functions. I am unsure why my arrow functions required a change in syntax depending upon whether or not I used curly braces. If I omitted curly braces but included the keyword return, I got a undefined error message. Can somebody explain why return is necessary when curly braces are present, but not when they are omitted?
Below are the various functions that seemed to work for me:
As a function declaration
function getRandomNumber(lower, upper) {
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
console.log(getRandomNumber( 1, 20));
Rewritten as a function expression
const getRandomNumber = function(lower, upper) {
return Math.floor(Math.random() * (upper - lower + 1)) + lower
};
console.log(getRandomNumber(1, 20));
Rewritten as an Arrow Function - multiple lines and including curly braces
const getRandomNumber = (lower, upper) => {
return Math.floor(Math.random() * (upper - lower + 1)) + lower
};
concole.log(getRandomNumber(1, 20));
Rewritten as an Arrow Function - more concise and including curly braces
const getRandomNumber = (lower, upper) => {return Math.floor(Math.random() * (upper - lower + 1)) + lower};
concole.log(getRandomNumber(1, 20));
Rewritten as an Arrow Function - most concise, omitting curly braces
const getRandomNumber = (lower, upper) => Math.floor(Math.random() * (upper - lower + 1)) + lower;
concole.log(getRandomNumber(1, 20));
1 Answer
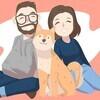
Clinton Hays
Front End Web Development Techdegree Graduate 18,156 PointsHi Rachael,
Doak is right. If you use curly braces, javascript is looking for a code block. Meaning you are going to tell it explicitly what needs to be returned. If you omit the curly braces, javascript is simply running through the code you have specified. The expression on the right side of => is implicitly returned. That's why it only works if there is only one line of code to run. As you already know, your 4th snippet doesn't require the curly braces or return statement, but take this code for example:
const randNum = () => {
const lower = prompt('Choose a lower limit for your range...');
const upper = prompt('Choose an upper limit for your range...');
const num = Math.floor(Math.random() * (+upper - +lower + 1)) + +lower;
return (document.querySelector('main').innerHTML = `<h1>${num}</h1>`);
};
Since the expression after the => is four lines, we must include the curly braces and thus a return statement.
I found this article to be pretty helpful. It seems like you've already got it down, though. Keep it up and happy coding! :)
Doak Covington
4,644 PointsDoak Covington
4,644 PointsWith the "concise body" the expression becomes the implicit return value.
With the "block body" you must be explicit in what is returned, thus the need of the return keyword.
Source: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Functions/Arrow_functions