Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial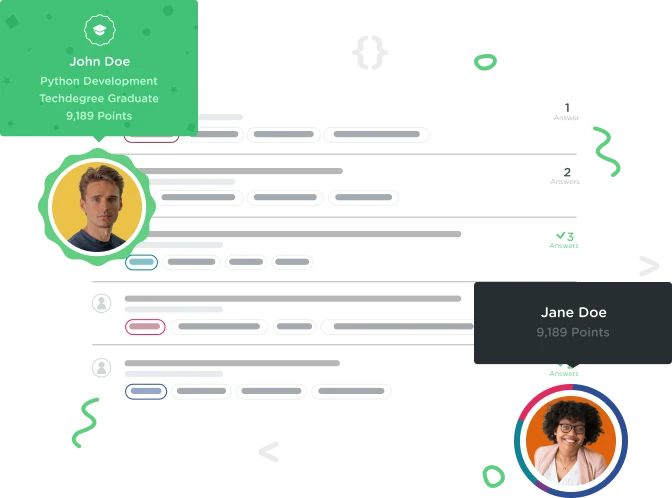
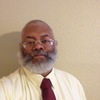
Adiv Abramson
6,919 PointsWhy is the sort() a static method instead of an instance method?
Why do we call the sort method off of the Arrays class instead of off of an array object, like myarray.sort()?
Also, is there a way to reset an array variable so that it has zero elements? Is there a way to destroy it so that myarray == null?
Strings are immutable in Java and so are arrays. If so, then why is the following operation legal:
mystring = "ABC"; mystring += "DEF";
yet the following operation is not
String[] myarray = {"A", "B", "C"}; myarray += "D";
If both data types are immutable shouldn't String reference types be subjected to the same type of workarounds that array reference types are? If with Strings Java creates a new object and assigns it to the reference, leaving the original object available for garbage collection, why doesn't it do that for arrays as well?
Thanks
1 Answer

Danial Goodwin
13,247 PointsBeing mutable or immutable just means if an object can change or not; It's not about using any operators.
Basically, the reason you can't do that operation on arrays is because of the language design. Strings were designed to be "added" together, and arrays weren't. (In some other languages, it is possible to use the plus sign to append to arrays.) Another way to put it, String
s are more of a "first-class citizen" for Java whereas Array
s are not.
The same "language design" reason applies to why Java uses static Arrays.sort(myArray)
instead of instance of myArray.sort()
. Different language designers choose to design languages in different ways. One possible reason for the Java designers is that sort()
isn't always necessary for arrays so they didn't want to force developers to always make sure that it is accurately implemented.
To "destroy" an array in Java, you just have to set it to null, like myArray = null
. Then, once the variable falls out of scope, it will be garbage collected. To make a basic array variable have zero elements, you can either iterate through all and set each element to null. Or, you can just assign the array variable to a new array variable and have the old array garbage collected. If you are using a Collection
, like List
or ArrayList
, then you can do (myArrayList.clear())[https://docs.oracle.com/javase/8/docs/api/java/util/ArrayList.html#clear--]
to remove all elements.