Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial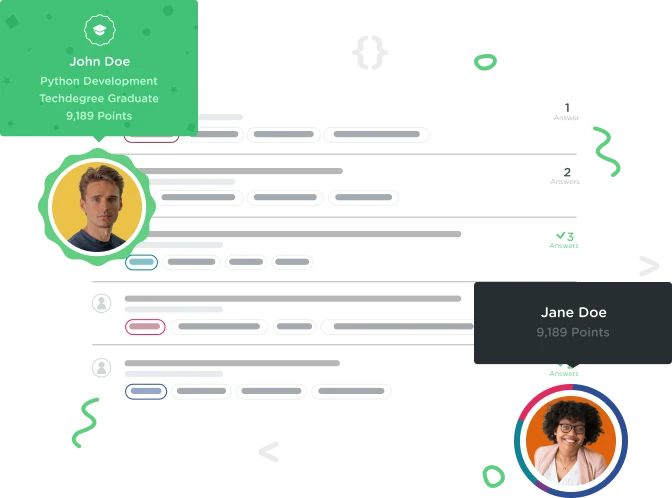

Kameron Keller
1,764 PointsWhy is the variable message initialized with a blank string, while the variable search is not?
var message = '';
var student;
var search;
When I completed the last challenge, I had message initialized as:
var message;
This caused the first entry on my HTML document to be undefined. I'm not sure I understand when to or not to initialize a variable with a blank string.
1 Answer
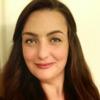
Jennifer Nordell
Treehouse TeacherHi there! Whenever you create a variable in JavaScript without initializing it with a value, it's type is undefined. JavaScript can be pretty smart. It has something called type inferencing. In many cases, it can determine what kind of data a variable is supposed to hold based on how you use it later.
This is all fine and dandy until we get to addition/concatenation. Without message
holding an initial value, if you were to use message += "something";
it will not know how to handle that. It cannot concatenate or add to an undefined
value. But when assigning an initial value, it can figure that out.
Run this in your browser console and take a look:
var thing1 = 1;
var thing2 = "1";
var thing3;
console.log(thing1 + 2);
console.log(thing2 + 2);
console.log(thing3 + 2);
console.log(thing3 += thing2);
The first line prints 3, which should be self explanatory. The second line however, prints 12. Because it's converting the 2 into a string to match the "1". The third line line prints NaN because this is what happens when you try to add something to an undefined
. And the last one prints undefined1, because you're concatenating a 1 onto the end of undefined
.
The bottom line is, if you know a variable is going to be holding a string that you will later be concatenating onto, you should initialize it with an empty string so that JavaScript is aware that this is a string and can reasonably use the concatenation operator on it. Personally, I initialize all my variables that I know will be strings with empty strings just to be on the safe side
Hope this helps!