Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial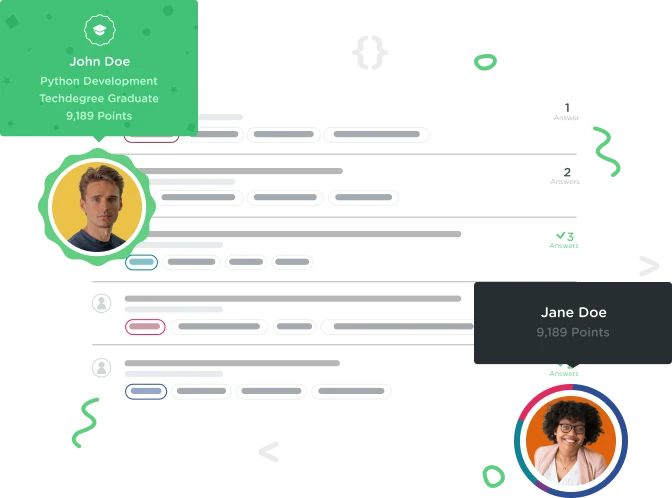

Babatunde Obidare
Courses Plus Student 10,514 PointsWhy is there no C# example?
I'm disappointed that there is no C# code here!!!
2 Answers
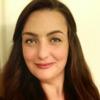
Jennifer Nordell
Treehouse TeacherHi there! I feel like that's a typo. I feel like the section marked as "C" should have been marked as "C#" given that it uses classes/objects found in the C# language. ANSI C is not object-oriented.
Hope this helps!

Kiron Roy
3,210 PointsHere is a link to a simple linear search in C#. All the logic is written in the main function (which is not advisable), but one can see what is going easier. Raw code below.
using System;
// Source: https://www.c-sharpcorner.com/blogs/linear-search-in-console-application1
namespace LinearSearch
{
class Program
{
static void Main()
{
var numberArray = new int[100];
Console.WriteLine("Enter the number of elements");
string userInputElements = Console.ReadLine();
int usersNumbers = Int32.Parse(userInputElements);
Console.WriteLine("---------------------------");
Console.WriteLine("\n Enter the array elements. Press enter/return after each number \n");
for (int i = 0; i < usersNumbers; i++)
{
string usersInputNumbers = Console.ReadLine();
numberArray[i] = Int32.Parse(usersInputNumbers);
}
Console.WriteLine("---------------------------");
Console.WriteLine("Enter the Search element\n");
string usersSearchElement = Console.ReadLine();
int usersSearchNumber = Int32.Parse(usersSearchElement);
for (int i = 0; i < usersNumbers; i++)
{
if (numberArray[i] == usersSearchNumber)
{
Console.WriteLine("---------------------------");
Console.WriteLine("Search successful!");
Console.WriteLine("Element {0} found at location {1}\n", usersSearchNumber, i + 1);
Console.ReadLine();
}
}
Console.WriteLine("Entered element not found. Search unsuccesful.");
Console.ReadLine();
}
}
}
Babatunde Obidare
Courses Plus Student 10,514 PointsBabatunde Obidare
Courses Plus Student 10,514 PointsThanks!
Pasan Premaratne
Treehouse TeacherPasan Premaratne
Treehouse TeacherWhoops! It was marked C# but turns out its stripped out of the resulting Markdown. Who knew!