Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial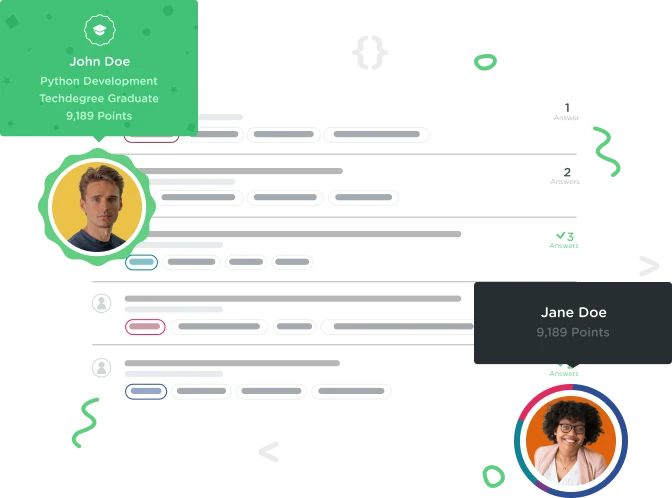
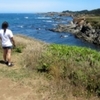
Nancy Melucci
Courses Plus Student 35,157 PointsWhy is this an infinite loop (Python)?
This is my second question today. And this one is really making me feel dumb. If someone could look at the code for this calculator in Python, and tell me why it produces an infinite loop (because I can't see why the "goodbye" statement doesn't happen if the func = "X'
I would be grateful. For a bonus, if I could make the string comparison case insensitive, that would make my day BUT really I need to know why this is throwing an infinite loop. Thanks.
import math
def rad2deg(value):
if 0 <= value <= 6.28319:
degrees = value * (180 / math.pi)
elif -6.28319 <= value <= 0:
degrees = value * (180 / math.pi) * -1
elif value > 6.28319:
value = value % 6.28319
degrees = value * (180 / math.pi)
elif value < -6.28319:
value = value % 6.28319
degrees = value * (180 / math.pi)
return degrees
print('This calculator requires you to enter a function and a value.\n')
print('The functions are as follows')
print('S - Sine')
print('C - Cosine')
print('T - Tangent')
print('R - Square Root')
print('N - Natural Log')
print('X - eXit the program')
functionValue = input("Please enter a function and a value: ")
func, value = functionValue.split(' ')
value = float(value)
degValue = math.sin(rad2deg(value))
while func is not 'X':
if func == 'S':
print("The sine of your number is " + str(math.sin(value)))
print("The sine of your number in degrees is " + str(rad2deg(math.sin(value))))
elif func == 'C':
print("The cosine of your number is " + str(math.cos(value)))
print("The sine of your number in degrees is " + str(rad2deg(math.cos(value))))
elif func == 'T':
print("The tangent of your number is " + str(math.tan(value)))
print("The sine of your number in degrees is " + str(rad2deg(math.tan(value))))
elif func == 'R':
print("The square root of your number is " + str(math.sqrt(value)))
elif func == 'L':
print("The natural log of your number is " + str(math.log(value)))
else:
print("Please enter a valid function.")
print("Thank you for using the calculator")
print("Press any key to continue")
3 Answers

Jeff Wilton
16,646 PointsHi Nancy, Your program is in an infinite loop because the choice to enter a new function and value is Outside of your While loop, so the value that the While loop is evaluating will never change. In regards to your bonus question, one of ways to do a case-insensitive comparison is to simply force an input string to be converted to all upper or lower case.
Here is a working version of your program, though there are still a few kinks to work out if you want to make it non-breakable:
import math
def rad2deg(value):
if 0 <= value <= 6.28319:
degrees = value * (180 / math.pi)
elif -6.28319 <= value <= 0:
degrees = value * (180 / math.pi) * -1
elif value > 6.28319:
value = value % 6.28319
degrees = value * (180 / math.pi)
elif value < -6.28319:
value = value % 6.28319
degrees = value * (180 / math.pi)
return degrees
print('This calculator requires you to enter a function and a value.\n')
print('The functions are as follows')
print('S - Sine')
print('C - Cosine')
print('T - Tangent')
print('R - Square Root')
print('N - Natural Log')
print('X - eXit the program')
func = ''
while func is not 'X':
functionValue = input("Please enter a function and a value: ").upper()
if functionValue == 'X':
print("Thank you for using the calculator")
any_key = input("Press any key to continue")
break
func, value = functionValue.split(' ')
value = float(value)
degValue = math.sin(rad2deg(value))
if func == 'S':
print("The sine of your number is " + str(math.sin(value)))
print("The sine of your number in degrees is " + str(rad2deg(math.sin(value))))
elif func == 'C':
print("The cosine of your number is " + str(math.cos(value)))
print("The sine of your number in degrees is " + str(rad2deg(math.cos(value))))
elif func == 'T':
print("The tangent of your number is " + str(math.tan(value)))
print("The sine of your number in degrees is " + str(rad2deg(math.tan(value))))
elif func == 'R':
print("The square root of your number is " + str(math.sqrt(value)))
elif func == 'L':
print("The natural log of your number is " + str(math.log(value)))
else:
print("Please enter a valid function.")
If there are any further questions, feel free to ask - and happy coding!

Thomas Nilsen
14,957 PointsBecause you only offer the user the ability to give a command and a number once. Meaning that once we start the while-loop it will just keep going.
Add this at the end of you while loop:
functionValue = input("Please enter a function and a value: ")
Also, It doesn't make much sense to having to enter X and a number in order to quit. Just X should be enough.
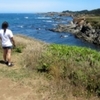
Nancy Melucci
Courses Plus Student 35,157 PointsThe professor's code has the X and a 0, which I did not understand either. NJM
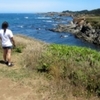
Nancy Melucci
Courses Plus Student 35,157 PointsThank you so much.
I hope I can remember that next time.
I guess if I make the mistake a few more times, it will stick.
Nancy M.

Jeff Wilton
16,646 PointsThat which does not work the first time makes you a stronger coder. :)