Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial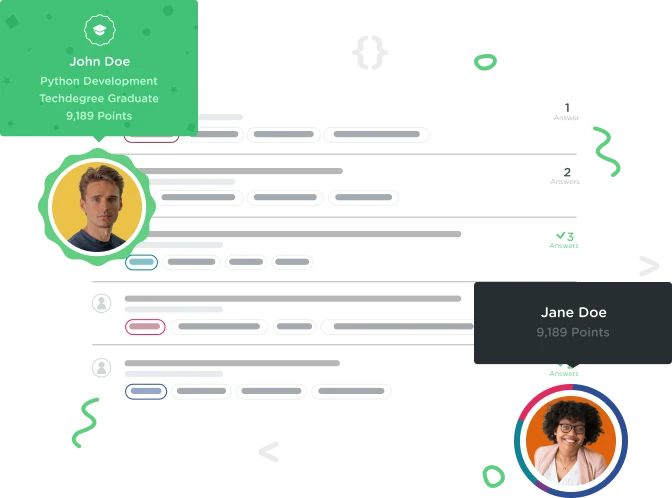
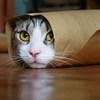
Herman Brummer
6,414 PointsWhy is this flopping?
list = [1, 2, 3, 4, 5]
def reverse_evens(iterable):
list = iterable[::2]
list = sorted(list)
return list[::-1]
print reverse_evens(list)
get this error # Bummer! Didn't get the right values from reverse_evens
.
These are the instructions:
You're on fire! Last one and it is, of course, the hardest. Make a function named reverse_evens that accepts a single iterable as an argument. Return every item in the iterable with an even index...in reverse. For example, with [1, 2, 3, 4, 5] as the input, the function would return [5, 3, 1]. You can do it!
3 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Herman,
You don't have to sort the list. You only need to get a slice of the even indexes and then another slice to reverse it.
You can also do this in one step if you'd like: return iterable[::2][::-1]
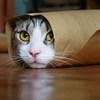
Herman Brummer
6,414 PointsThank you Jason, but why does that even work?!
I get the iterable[::2] part
which is basically start, stop, step, so from start to end and then step is == 2
but [::-1] that you added on... huh... what on earth is that?

Jason Anello
Courses Plus Student 94,610 PointsIt's the same code that you have, minus the sorted function, but it's combined into one step.
So the first part that you get is getting all the even indexes.
Then the [::-1]
slice is reversing it with a -1 step.
It's the same as this:
def reverse_evens(iterable):
list = iterable[::2]
return list[::-1]
except instead of assigning the first slice to a variable and then reversing it in a second step, you can do both slices in one step.

Douglas Brunson
Courses Plus Student 2,458 PointsI tried out your code and I do get [5,3,1] as the output. It works for other lists as well.
But it looks like it does not work for strings. If I input a string like "12345", the output is the list ['5','3','1'] when it should actually be the string '531'. I think this is because you used the sorted function, which will create a list of your iterable in ascending order. Hope this makes sense.

Jason Anello
Courses Plus Student 94,610 PointsHi Douglas,
It would work out for any list that starts out as being sorted already like the list in the example. This is because the sorted method will return back the same list since it's already sorted. But if you had a list of unsorted numbers then you wouldn't get back the right results.

Piotr Andrzejewski
1,145 PointsHi
I've reached the same problem. My solutions is:
def reverse_evens(iterable):
return iterable[::-2]
If you try to test the function you are given the expected results.
print(reverse_evens([1, 2, 3, 4, 5]))
# results : [5, 3, 1]
I really don't know why I can't pass this exercise even if the results produced by my func are identical to expected ones.
Maybe I did sth wrong?

Ismail Khan
1,023 PointsI'm having the exact same problem as well.
Herman Brummer
6,414 PointsHerman Brummer
6,414 PointsI get the correct output