Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial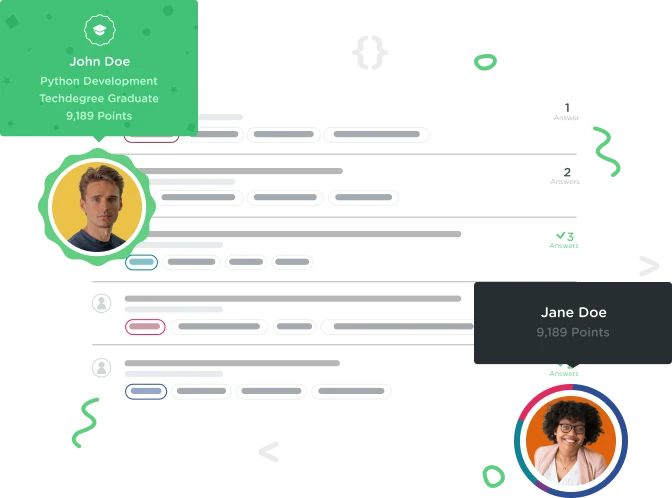
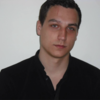
Eli Tamosauskas
9,776 PointsWhy is this not working
Why is this code not passing? Can anyone see?
<?php
class Fish {
public $common_name;
public $flavor;
public $record_weight;
public function __construct($name, $flavor, $record) {
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
//methods
public function getInfo () {
echo "A " . $name . " is an " . $flavor . " flavoured fish. The world record weight is " . $weight . ".";
};
}
$bass = new Fish ("Largemouth Bass", "Excellent", "22 punds 5 ounces");
getInfo($bass);
?>
3 Answers
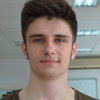
Vadim Zelenin
13,045 Pointsecho function should take a string. So just wrap your text after 'echo' into a ' ' or " "
upd: you should call 'getInfo' as a method of the object called $bass like: $bass->getInfo();
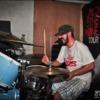
Mike Costa
Courses Plus Student 26,362 PointsHey Eli,
There's a couple things that are going on here as to why your code is not passing. I'll try to explain classes the best way I can to see if you can figure out why your code doesn't work.
We'll start with this Fish class example and only use 1 property for the class, "common name".
<?php
class Fish {
public $common_name;
}
This is giving the class Fish a property called $common_name. Now we'll add the construct method:
<?php
class Fish {
public $common_name;
public function __construct($name) {
$this->common_name = $name;
}
}
The construct method runs once you create a new instance of the class. Now lets create a 2 new instances of this class.
<?php
class Fish {
public $common_name;
public function __construct($name) {
$this->common_name = $name;
}
}
$fish_one = new Fish("Eli");
$fish_two = new Fish("Mike");
We now have TWO fish objects, each with different names. Notice how I created the objects outside of the class. If you want to access the property of $common_name on either object, you can call it based off of the object you created.
echo $fish_one->common_name; // Outputs: "Eli"
echo $fish_two->common_name; // Outputs: "Mike"
If you want to create a method in the class that returns the name for you, you would use the $this keyword inside of the class and its methods. Let's create 2 methods called getCommonName and greeting and then see how they're used.
<?php
class Fish {
public $common_name;
public function __construct($name) {
$this->common_name = $name;
}
public function getCommonName(){
return $this->common_name;
}
public function greeting(){
return "Good day, " . $this->common_name . "!";
}
}
$fish_one = new Fish("Eli");
$fish_two = new Fish("Mike");
echo $fish_one->getCommonName(); // Outputs: "Eli"
echo $fish_one->greeting(); // Outputs: "Good day, Eli!"
echo $fish_two->getCommonName(); // Outputs: "Mike"
echo $fish_two->greeting(); // Outputs: "Good day, Mike!"
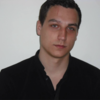
Eli Tamosauskas
9,776 PointsThats a very nice explanation, however that didnt solve my code problem. I understood the concept just fine i think. Code still not passing though
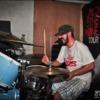
Mike Costa
Courses Plus Student 26,362 PointsEli Tamosauskas Would you mind posting your new modified code here so I can see where its going wrong?
Eli Tamosauskas
9,776 PointsEli Tamosauskas
9,776 PointsBut my echo text IS wrapped, look at example above? Also tried your method calling didnt solve.