Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial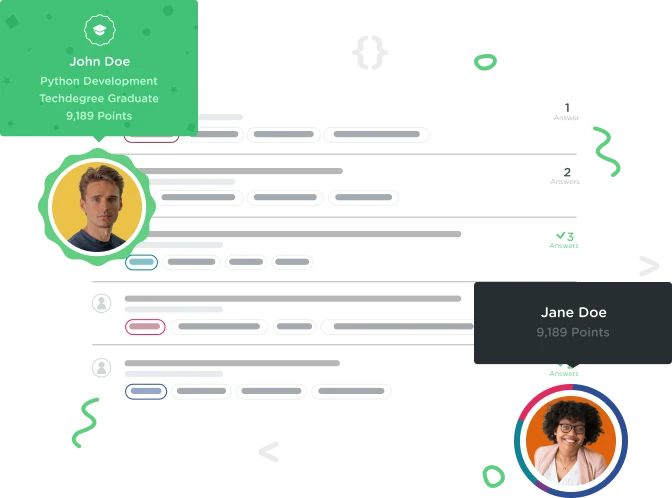
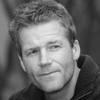
Maarten De Kruif
7,314 PointsWhy is this not working??
I don't have a clue how to solve this question. Any hints? Thanks in advance!
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
import com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
String result = "";
if (result instanceof Object)
result = (String) obj;
return result;
if (obj instanceof BlogPost)
result = String.valueOf(bp);
return result;
}
}
1 Answer
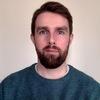
Richard Lambert
Courses Plus Student 7,180 PointsHello mate,
1st Problem
From Code Conventions for the Java Programming Language:
Braces are used around all statements, even single statements, when they are part of a control structure, such as an if-else or for statement. This makes it easier to add statements without accidentally introducing bugs due to forgetting to add braces.
and
Note: if statements always use braces, {}. Avoid the following error-prone form:
if (condition) //AVOID! THIS OMITS THE BRACES {}! statement;
Multiple statements, that are to be executed when an if statement's conditional expression is satisfied, are always enclosed in braces. It's possible to omit the braces if the code to be executed is a single statement, but this is considered bad practice.
2nd Problem
An instance of any class can be passed as the argument to getTitleFromObject(Object obj)
since its parameter is of type Object
and all classes inherit from this. For this task, you're interested in checking whether the object passed in is either of type String
or BlogPost
, hence:
if (obj instanceof String) {
//assign string instance to result
} else if (obj instanceof BlogPost) {
//get blogpost title and assign to result
}
You correctly declare and explicitly initialize String result = "";
outside of and prior to your if statements, ensuring it remains visible within the entire method body. This enables you to assign a value to this variable from within your if-statements, but also return the object referenced at the end of the method body:
String result = "";
if (obj instanceof String) {
//assign string instance to result
} else if (obj instanceof BlogPost) {
//get blogpost title and assign to result
}
return result;
Now for the type-casting. As far as the compiler is concerned, it cannot make any assumptions as to the type of object being passed as an argument to getTitleFromObject(Object obj)
other than that it's definitely of type Object
. As far as it's concerned, the object passed as the argument is a generic Object
, possessing only properties and behavior that the Object
class defines. Having checked you have an instance of a more specific class, access to its more specific functionality is achieved through type-casting (downcasting in this case):
String result = "";
if (obj instanceof String) {
//If this code block is entered you know obj is a String. Downcast from Object to String.
result = (String) obj;
} else if (obj instanceof BlogPost) {
//Similarly, if this code block is entered you know obj is a BlogPost. Downcast from Object to BlogPost.
BlogPost blogPost = (BlogPost) obj;
//By downcasting to BlogPost, you now have access to the methods which this class defines.
result = //Which method are you going to use to complete this task???
}
return result;
Hope this helps
Maarten De Kruif
7,314 PointsMaarten De Kruif
7,314 PointsThanks a lot Richard with this extensive explanation!