Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial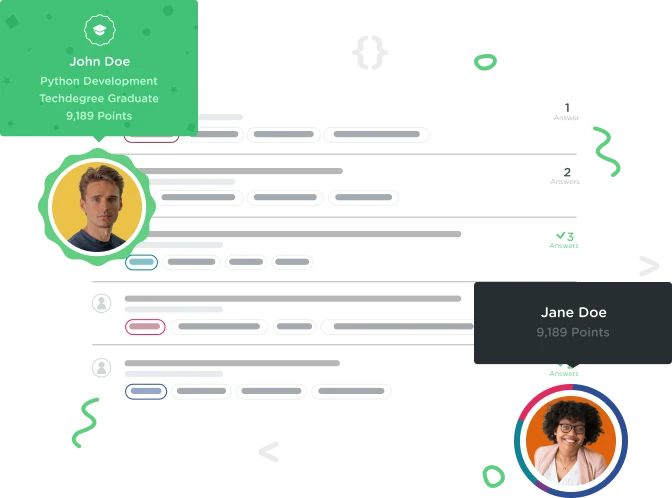

James Dunn
3,041 PointsWhy is this producing the wrong result?
Once again I'm lost. I know for a fact that stats() produces the a list of lists. And that the lists are formatted as such [teacher_name, number_of_courses], as is required by the challenge.
However, when I plug it into the challenge it says that it isn't producing the correct results.
Any help would be much appreciated.
Thank you.
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(my_dict):
num_of_teachers = 0
for key in my_dict:
num_of_teachers += 1
return num_of_teachers
#End of num_teachers
def num_courses(my_dict):
num_of_courses = 0
for value in my_dict.values():
for item in value:
num_of_courses += 1
return num_of_courses
def courses(my_dict):
course_list = []
for value in my_dict.values():
for item in value:
course_list.append(item)
return course_list
def most_courses(my_dict):
answer = ""
max_val = 0
dict_items = None
teacher = ""
i = 0
for key in my_dict.keys():
for item in my_dict.values():
dict_items = item
for value in dict_items:
i += 1
if i > max_val:
max_val = i
teacher = key
elif i == max_val:
teacher = teacher + "&" + key
return teacher
def stats(my_dict):
answer = []
stat = []
course_cnt = 0
for key in my_dict.keys():
for course in my_dict.values():
course_cnt += 1
stat = [key, course_cnt]
course_cnt = 0
answer.extend(stat)
return answer
2 Answers
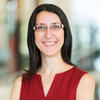
Louise St. Germain
19,424 PointsHi James,
You're very close! There are two minor things to fix, and then it should work properly.
First, for this line:
for course in my_dict.values():
my_dict.values() contains a list of lists (i.e., a list of all the values, which are themselves lists), so while you're expecting it to be looping through all the items in the list that goes with that key, what it's actually doing is looping through its higher-level list, i.e., at the teacher level. So essentially it's counting the total number of teachers, not the total number of courses for a given teacher.
If you change that line to this, it should work properly:
for course in my_dict[key]:
Second, you correctly create a list with the teacher and their stat, but when you extend the answer list, it creates one big continuous list, instead of a list of lists. Your code is outputting something like,
['Kenneth Love', 3, 'Andrew Chalkley', 2, 'Craig Dennis', 5]
instead of:
[['Kenneth Love', 3], ['Andrew Chalkley', 2], ['Craig Dennis', 5]]
If you use append instead of extend, that should fix the problem.
answer.append(stat)
With those two little things, I think you should be able to finish the challenge. I hope this helps!

James Dunn
3,041 PointsThanks! That took care of the problem.
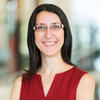
Louise St. Germain
19,424 PointsGreat! Glad it worked out. :-)