Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial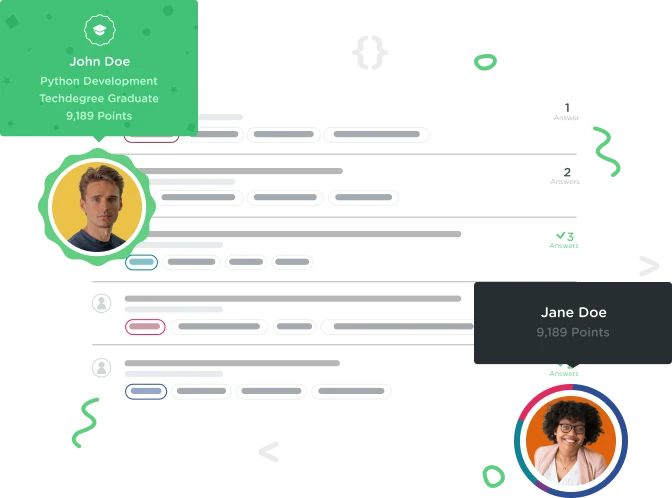

Susan Rusie
10,671 PointsWhy is this saying there is a syntax error?
I am trying to figure out what could be wrong with my code. I assume that the idea for the extra credit is for the text for the css to print to the console when I run Node but it is saying there is an syntax error with this for the following code because it won't run Node:
function user(request, response){
Here is my code for the router.js:
var Profile = require("./profile.js");
var renderer = require("./renderer.js");
var querystring = require("querystring");
var fs = require('fs');
var commonHeaders = {'Content-Type': 'text/html'};
var commonHeaders = {'Content-Type': 'text/css'};
//function that reads css
function css(request, response) {
//if url == "/" && GET
if(request.url.indexOf === (".css") !== -1){
var file = fs.readFileSync(`.${request.url}`, {'encoding' : 'utf8'});
response.writeHead(200, {'Content-Type' : 'text/css'});
response.write(file);
request.end();
}
}
if(request.method.toLowerCase() === "get") {
//show search
response.writeHead(200, cssHeaders);
renderer.css(response);
response.writeHead(200, commonHeaders);
renderer.view("header", {}, response);
renderer.view("search", {}, response);
renderer.view("footer", {}, response);
response.end();
} else {
//if url == "/" && POST
//redirect to /: username
//get the post data from body
request.on("data", function(postBody) {
//extract the username
var query = querystring.parse(postBody.toString());
//redirect to username/:
response.writeHead(303, {"Location": "/" + query.username});
response.end();
});
}
}
//Handle HTTP route GET /:username i.e. /chalkers
function user(request, response){
//if url == "/...."
var username = request.url.replace("/", "");
if(username.length > 0 && request.url.indexOf('.css') === -1){
response.writeHead(200, {'Content-Type', : 'text/css'});
response.write(username);
response.end();
}
}
//get json from Treehouse
var studentProfile = new Profile(username);
//on "end"
studentProfile.on("end", function(profileJSON){
//show profile
//Store the values which we need
var values = {
avatarUrl: profileJSON.gravatar_url,
username: profileJSON.profile_name,
badges: profileJSON.badges.length,
javascriptPoints: profileJSON.points.JavaScript
}
//Simple response
renderer.view("profile", values, response);
renderer.view("footer", {}, response);
response.end();
});
//on "error"
studentProfile.on("error", function(error){
//show error
renderer.view("error", {errorMessage: error.message}, response);
renderer.view("search", {}, response);
renderer.view("footer", {}, response);
response.end();
});
}
}
module.exports.home = home;
module.exports.user = user;
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<title>Treehouse Profile</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style type="text/css" media="screen">
<!-- link contains actual path to css -->
<link rel="stylesheet" type="text/css" href="./styles/main.css" media="screen">
</head>
Here is my error.html (in the designs folder):
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<title>Treehouse Profile</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style type="text/css" media="screen">
@import url(http://fonts.googleapis.com/css?family=Varela+Round);
@import url(http://necolas.github.io/normalize.css/3.0.2/normalize.css);
body {
background: #ECEEEF;
text-align: center;
margin: 100px auto;
max-width: 462px;
padding: 0 40px;
font: 18px normal 'Varela Round', Helvetica, serif;
color: #777B7E;
}
img {
width: 100%;
}
#searchIcon, #avatar {
width: 50%;
border-radius: 50%;
margin: 0 25% 0 25%;
}
input {
font-family: 'Varela Round', Helvetica, serif;
font-size: 18px;
padding: 31px 0;
margin: 10px 0;
text-align: center;
width: 360px;
border-radius: 4px;
border: 1px solid #D5DDE4;
color: #2C3238;
}
#username {
margin: 20px 0 0;
}
.button {
border-color: #5FB6E1;
background: #5FB6E1;
color: #fff;
}
#error {
width: 100%;
padding: 22px 0;
background: #FFE6B2;
color: #C5A14E;
position: absolute;
left: 0;
top: 0;
}
#profile {
background: #fff;
border-radius: 4px;
border: 1px solid #D5DDE4;
padding: 40px 0 0;
margin: -40px 0 0;
}
ul {
list-style-type: none;
padding: 0;
margin: 40px 0 0;
}
li {
display: inline-block;
width: 100%;
padding: 22px 0;
margin: 0;
border-top: 1px solid #D5DDE4;
}
a, a:visited {
color: #5FB6E1;
text-decoration: none;
}
span {
color: #2C3238;
}
</style>
</head>
<body><div id="error">There was an error getting the profile for new. (Not Found)</div><img src="http://i.imgur.com/VKKm0pn.png" alt="Magnifying Glass" id="searchIcon">
<form action="/" method="POST">
<input type="text" placeholder="Enter a Treehouse username" id="username" name="username">
<input type="submit" value="submit" class="button">
</form></body>
</html>
Here is my profile.html (in the designs folder):
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<title>Treehouse Profile</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style type="text/css" media="screen">
@import url(http://fonts.googleapis.com/css?family=Varela+Round);
@import url(http://necolas.github.io/normalize.css/3.0.2/normalize.css);
body {
background: #ECEEEF;
text-align: center;
margin: 100px auto;
max-width: 462px;
padding: 0 40px;
font: 18px normal 'Varela Round', Helvetica, serif;
color: #777B7E;
}
img {
width: 100%;
}
#searchIcon, #avatar {
width: 50%;
border-radius: 50%;
margin: 0 25% 0 25%;
}
input {
font-family: 'Varela Round', Helvetica, serif;
font-size: 18px;
padding: 31px 0;
margin: 10px 0;
text-align: center;
width: 360px;
border-radius: 4px;
border: 1px solid #D5DDE4;
color: #2C3238;
}
#username {
margin: 20px 0 0;
}
.button {
border-color: #5FB6E1;
background: #5FB6E1;
color: #fff;
}
#error {
width: 100%;
padding: 22px 0;
background: #FFE6B2;
color: #C5A14E;
position: absolute;
left: 0;
top: 0;
}
#profile {
background: #fff;
border-radius: 4px;
border: 1px solid #D5DDE4;
padding: 40px 0 0;
margin: -40px 0 0;
}
ul {
list-style-type: none;
padding: 0;
margin: 40px 0 0;
}
li {
display: inline-block;
width: 100%;
padding: 22px 0;
margin: 0;
border-top: 1px solid #D5DDE4;
}
a, a:visited {
color: #5FB6E1;
text-decoration: none;
}
span {
color: #2C3238;
}
</style>
</head>
<body><div id="profile">
<img src="http://i.imgur.com/KMi72wU.png" alt="Avatar" id="avatar">
<p><span>@waldo</span></p>
<ul>
<li><span>154</span> Badges earned</li>
<li><span>652</span> JavaScript points</li>
<li><a href="/">search again</a></li>
</ul>
</div></body>
</html>
Here is my error.html(in the views folder):
<div id="error">{{errorMessage}}</div>
Here is my footer.html(in the views folder):
</body>
</html>
Here is my header.html (in the views folder):
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<title>Treehouse Profile</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style type="text/css" media="screen
<link rel="stylesheet" href="styles.css"/>
@import url(http://fonts.googleapis.com/css?family=Varela+Round);
@import url(http://necolas.github.io/normalize.css/3.0.2/normalize.css);
body {
background: #ECEEEF;
text-align: center;
margin: 100px auto;
max-width: 462px;
padding: 0 40px;
font: 18px normal 'Varela Round', Helvetica, serif;
color: #777B7E;
}
img {
width: 100%;
}
#searchIcon, #avatar {
width: 50%;
border-radius: 50%;
margin: 0 25% 0 25%;
}
input {
font-family: 'Varela Round', Helvetica, serif;
font-size: 18px;
padding: 31px 0;
margin: 10px 0;
text-align: center;
width: 360px;
border-radius: 4px;
border: 1px solid #D5DDE4;
color: #2C3238;
}
#username {
margin: 20px 0 0;
}
.button {
border-color: #5FB6E1;
background: #5FB6E1;
color: #fff;
}
#error {
width: 100%;
padding: 22px 0;
background: #FFE6B2;
color: #C5A14E;
position: absolute;
left: 0;
top: 0;
}
#profile {
background: #fff;
border-radius: 4px;
border: 1px solid #D5DDE4;
padding: 40px 0 0;
margin: -40px 0 0;
}
ul {
list-style-type: none;
padding: 0;
margin: 40px 0 0;
}
li {
display: inline-block;
width: 100%;
padding: 22px 0;
margin: 0;
border-top: 1px solid #D5DDE4;
}
a, a:visited {
color: #5FB6E1;
text-decoration: none;
}
span {
color: #2C3238;
}
</style>
</head>
<body>
Here is my profile.html (in the views folder):
<div id="profile">
<img src="{{avatarUrl}}" alt="Avatar" id="avatar">
<p><span>@{{username}}</span></p>
<ul>
<li><span>{{badges}}</span> Badges earned</li>
<li><span>{{javascriptPoints}}</span> JavaScript points</li>
<li><a href="/">search again</a></li>
</ul>
</div>
Here is my search.html (in the views folder):
<img src="http://i.imgur.com/VKKm0pn.png" alt="Magnifying Glass" id="searchIcon">
<form action="/" method="POST">
<input type="text" placeholder="Enter a Treehouse username" id="username" name="username">
<input type="submit" value="search" class="button">
</form>
Here is my app.js:
var router = require("./router.js");
//Problem: We need a simple way to look at a user's badge count and JavaScript point from a web browser
//Solution: Use Node.js to perform the profile look ups and server our template via HTTP
//Create a web server
var http = require('http');
http.createServer(function (request, response) {
if(request.url === "/") {
router.routeHome(request, response);
} else if(request.url.indexOf('.css') != -1) {
router.routeCss(request, response);
} else if(request.url.indexOf('.js') != -1) {
router.routeJs(request, response);
} else if(request.url.indexOf('.ico') != -1) {
//there is no favicon.ico at this time, do nothing
} else {
router.routeUser(request, response);
}
}).listen(3000);
console.log('Server running on port: ' + (process.env.PORT !== undefined ? process.env.PORT : 1337));
Here is my example_profile.js:
var Profile = require("./profile.js");
var studentProfile = new Profile("chalkers");
/**
* When the JSON body is fully recieved the
* the "end" event is triggered and the full body
* is given to the handler or callback
**/
studentProfile.on("end", console.dir);
/**
* If a parsing, network or HTTP error occurs an
* error object is passed in to the handler or callback
**/
studentProfile.on("error", console.error);
Here is my profile.js:
var EventEmitter = require("events").EventEmitter;
var https = require("https");
var util = require("util");
/**
* An EventEmitter to get a Treehouse students profile.
* @param username
* @constructor
*/
function Profile(username) {
EventEmitter.call(this);
profileEmitter = this;
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = https.get("https://teamtreehouse.com/" + username + ".json", function(response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
//Status Code Error
profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + ". [" + response.statusCode + response.statusMessage + "]"));
}
//Read the data
response.on('data', function (chunk) {
body += chunk;
profileEmitter.emit("data", chunk);
});
response.on('end', function () {
if(response.statusCode === 200) {
try {
//Parse the data
var profile = JSON.parse(body);
profileEmitter.emit("end", profile);
} catch (error) {
profileEmitter.emit("error", error);
}
}
}).on("error", function(error){
profileEmitter.emit("error", error);
});
});
}
util.inherits( Profile, EventEmitter );
module.exports = Profile;
Here is my renderer.js:
Here is var fs = require("fs");
function mergeValues(values, content) {
//Cycle over the keys
for(var key in values) {
//Replace all {{key}} with the value from the values object
content = content.replace("{{" + key + "}}", values[key]);
}
//return merged content
return content;
}
function css(response) {
var cssFile = fs.readFileSyn("./views/styles.css" + templateName + 'html', [encoding: "utf8"});
response.write(cssFile);
}
function view(templateName, values, reponse) {
//Read from the template file
var fileContents = fs.readFileSync('./views/' + templateName + '.html', {encoding: "utf8"});
//Insert values in to the content
fileContents = mergeValues(values, fileContents);
//Write out the contents to the response
reponse.write(fileContents);
}
module.exports.view = view;
Any help on this would be greatly appreciated.

Susan Rusie
10,671 PointsI am not really sure how to take a snapshot of all of my code since I am using Notepad++ instead of Workspaces. Do I need to create a zip file? If so, how do I do that? I am still pretty new to programming so any help you can offer will be greatly appreciated.
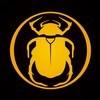
rydavim
18,813 PointsIn workspaces, you can do file > upload file
or drag and drop files in the left section to upload your work into workspaces.
You're also welcome to edit your original post, or post additional code blocks in the comments.
rydavim
18,813 Pointsrydavim
18,813 PointsI've been trying to work out what might be going wrong, but without all of your files it's proving difficult. Could you please post all of your code, or setup a workspace snapshot?
When I use your css code section with my own, I do not get any errors. Of course, the code is also not doing anything, because I don't have the rest of your files so I'm not sure how you're calling it elsewhere.
Hopefully we can get this working for you. :)