Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial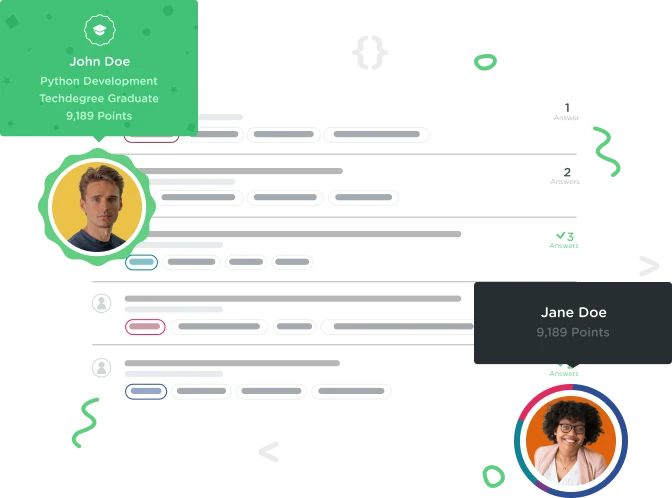
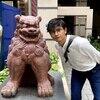
Carlos Chavez
5,691 PointsWhy is this script element not at the end of the body tag?
I noticed that the code Dave is writing has the script element outside the body. Does anybody know the reason for that? We have learned that best practice for placing the JS code is at the end of the body tag. And I have even experienced issues if with my code when I didn't follow this advice. This is why I was wondering about his positioning.
<head>
<meta charset="utf-8">
<link href='//fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<title>AJAX with JavaScript</title>
<script>
let xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (xhr.readyState === 4) {
document.getElementById('ajax').innerHTML = xhr.responseText;
}
};
xhr.open('GET', 'sidebar.html');
function sendAJAX() {
xhr.send();
document.getElementById('load').style.display = 'none';
}
</script>
</head>
<body>
<div class="grid-container centered">
<div class="grid-100">
<div class="contained">
<div class="grid-100">
<div class="heading">
<h1>Bring on the AJAX</h1>
<button id="load" onclick="sendAJAX()">Bring it to me!</button>
</div>
<div id="ajax">
</div>
</div>
</div>
</div>
</div>
</body>
2 Answers
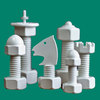
Steven Parker
241,488 PointsScripts that only load libraries, define functions, or establish data resources can be safely loaded in the "head" section of the page, and it is a common practice to do so.
But any script that interacts with the DOM should always be loaded as the last thing in the "body" section, and as you have seen can create errors if it is loaded before the page elements that it references.
This script is an example of the first kind since it defines functions that don't run until the page button is clicked.
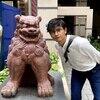
Carlos Chavez
5,691 PointsThank you Steven! I got confused for a second there. Sometimes I feel that I don't have full knowledge of where it's best to place some lines of code. But your explanation makes a lot of sense.