Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial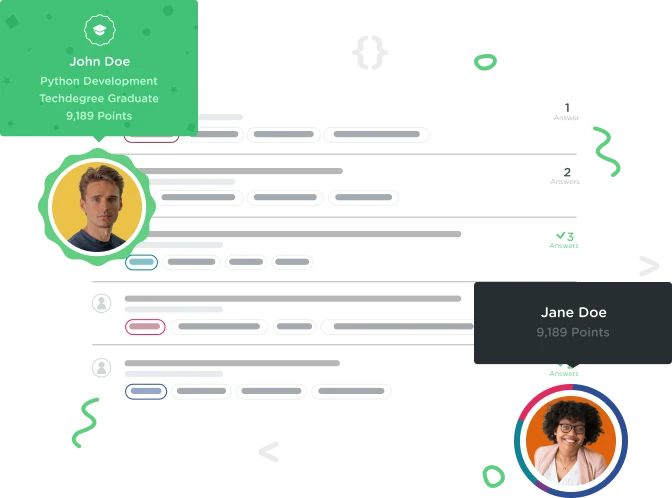

Matthew Cahn
6,301 PointsWhy is this showing a parse error?
Why is this showing a parse error?
<!DOCTYPE html>
<html lang="en">
<head>
<title> JavaScript Foundations: Objects</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Objects: Methods</h2>
<script>
var genericGreet = function() {
return "Hello, my name is " + this.name;
}
var andrew = {
name: "Andrew",
greet: genericGreet();
}
var ryan = {
name: "Ryan",
greet: genericGreet();
}
</script>
</body>
</html>
3 Answers
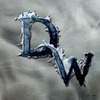
Hugo Paz
15,622 PointsHi Matthew,
You have a parse error due to the semi colons inside each object { greet:genericGreet(); }
If you remove them like this:
var genericGreet = function() {
return "Hello, my name is " + this.name;
};
var andrew = {
name: "Andrew",
greet: genericGreet
};
var ryan = {
name: "Ryan",
greet: genericGreet
};
You also dont need the parenthesis since you saved the function in the variable. Only when you call it do you need to use the parenthesis.
ryan.greet();

Matthew Cahn
6,301 PointsNo semicolons in objects got it. That's strange because he had semicolons in the video.
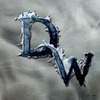
Hugo Paz
15,622 PointsIf you watch the video, you can see that he uses semicolons inside a function that is defined inside the object.
something like this:
{
name: 'andrew',
greet: function (){
console.log("Hi! My name is " + this.name);
}
}
when javascript looks inside the object literal, it does not expect a semicolon and throws an error.
Basically
var o = {
r: 'some value',
t: 'some other value'
};
is functionally equivalent to
var o = new Object();
o.r = 'some value';
o.t = 'some other value';

Matthew Cahn
6,301 PointsAhh, that makes sense.