Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial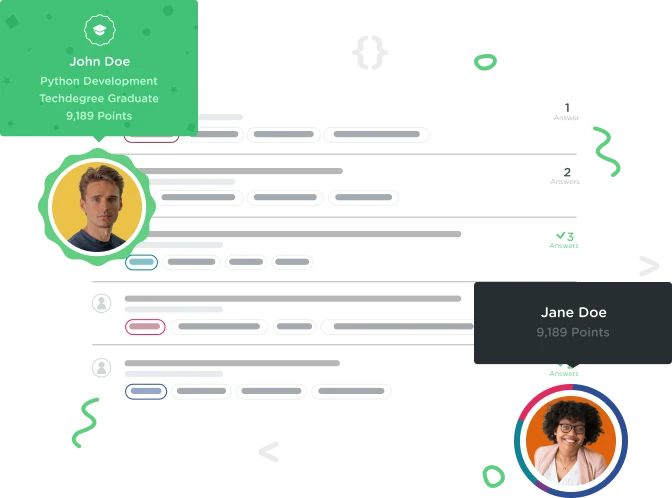

VICTORIA DENNIS
Full Stack JavaScript Techdegree Student 3,679 PointsWhy isn't it 4? What is a code block? Doesn't it run to "else" if no other condition is met?
I don't think I quite understand what a block is. But either way, why wouldn't it run all 4?
2 Answers
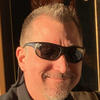
Peter Vann
36,427 PointsHi Victoria!
It's kind of a confusing question.
Consider this code:
let num = 3;
if (num === 0) {
// Do task "A"
} else if (num === 1) {
// Do task "B"
} else if (num === 2) {
// Do task "C"
} else { // Default - will only run if num is something other than 0, 1, or 2 - in this case, it's 3, so task "D" will run
// Do task "D"
}
A "block" is any code inside a set of curly braces.
The answer is 1 because only one block will ever run - in this case, only task "D" will run.
(In other words, it will skip tasks "A", "B", and "C")
If num was equal to 1, only task "B" would run (and the others would be skipped).
The only code that will run is the code in the block where the condition resolves to true.
Does that make sense now?
I hope that helps.
Stay safe and happy coding!
BTW, it could also be coded using a switch statement, like this:
let num = 3;
switch (num) {
case 0:
// Do task "A"
console.log("Task A");
console.log(num);
break;
case 1:
// Do task "B"
console.log("Task B");
console.log(num);
break;
case 2:
// Do task "C"
console.log("Task C");
console.log(num);
break;
default: // Any case that is NOT 0, 1, or 2
// Do task "D"
console.log("Task D");
console.log(num);
}
( You can test it by changing let num = )
Again, I hope that helps.
Stay safe and happy coding!

VICTORIA DENNIS
Full Stack JavaScript Techdegree Student 3,679 PointsYeah, I think I got caught up on the terminology. At the end of the lesson, I realized they were asking more along the lines of "how many actions would be executed" rather than codes read.