Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial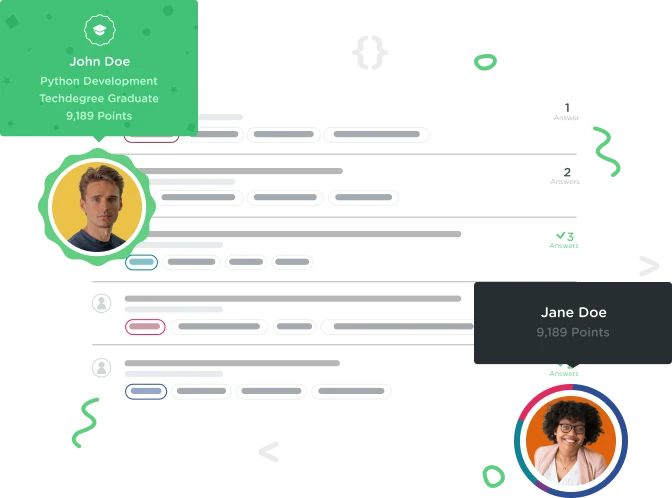
Jacob Brickell
8,083 PointsWhy isn't my answer being accepted? - Swift Generic Functions
This is the question:
Write a function named duplicate with a single generic type parameter T. The function takes two arguments, item of type T, and numberOfTimes of type Int, and returns an array of type T.
The function simply creates an array containing the element duplicated by the number of times specified. For example, calling duplicate(item: 1, numberOfTimes: 4) returns [1, 1, 1, 1].
my answer is this:
func duplicate<T>(item: inout T, numberOfTimes: Int) -> [T] { let newArray = Array(repeating: item, count: numberOfTimes) print(newArray)
return newArray
}
This works in a Swift playground but Treehouse is not accepting my answer. I've seen other ways of doing this but my answer works so I'm wondering if there is anything wrong with it.
func duplicate<T>(item: inout T, numberOfTimes: Int) -> [T] {
let newArray = Array(repeating: item, count: numberOfTimes)
print(newArray)
return newArray
}
1 Answer

andren
28,558 PointsThe issue is that you have made the item
an in-out parameter by using the inout
keyword. I don't know how you tested the code in Swift playground, but running your code like this: duplicate(item: 1, numberOfTimes: 4)
should not work in any Swift compiler. As in-out parameters do not accept literal or constant values.
Here is an online Swift REPL with your code loaded, if you try to run it the code will crash.
If you remove the inout
keyword like this:
func duplicate<T>(item: T, numberOfTimes: Int) -> [T] {
let newArray = Array(repeating: item, count: numberOfTimes)
return newArray
}
Then your code will work perfectly fine, and be accepted by the challenge.