Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial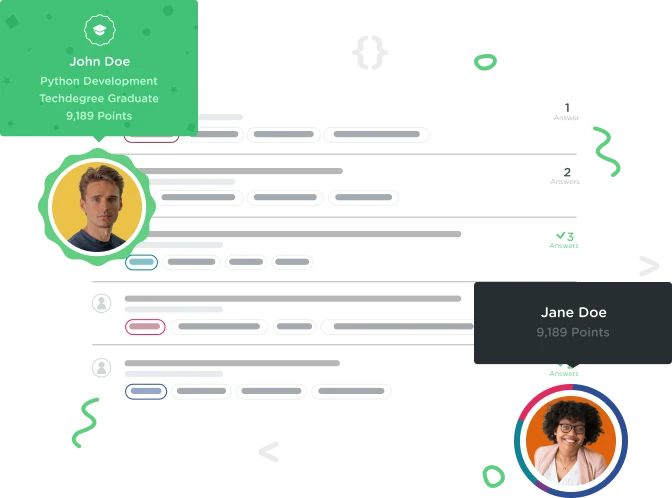

Adrian Satmarel
28,633 PointsWhy isn't my answer correct?
When I use the "Preview" it says "Killed" and I thought that it means it's OK.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
while(true)
{
try
{
Console.WriteLine("Enter the number of times to print \"Yay!\": ");
string input = Console.ReadLine();
if(input == null)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
else
{
int count = int.Parse(input);
if(count < 0)
{
Console.WriteLine("You must enter a positive number.");
continue;
}
else
{
int i = 0;
while(i < count)
{
i += 1;
Console.WriteLine("Yay!");
}
}
}
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
break;
}
}
}
}
3 Answers
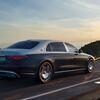
Balazs Peak
46,160 PointsYou have attempted to make tasks which are not demanded, for example, you've tried to validate the "wholeness" of the number with the first if statement. That is unneccessary because the parsing will take care of that. For example if you parse 3.11 to int, it will become 3 and so on.
Another example would be you have attempted to use continue and break keywords which I highly doubt would be applicable here. They are used with loops exclusively, as far as I'm concerned. If you want to make sure, you can look them up, but at this level it is not necessary.
This is one possible solution which, as you can see, might be a lot more simple than you might would have thought. Happy coding!
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
try{
Console.Write("Enter the number of times to print \"Yay!\": ");
string input = Console.ReadLine();
int count = int.Parse(input);
if(count >= 0){
int i = 0;
while(i < count)
{
i += 1;
Console.WriteLine("Yay!");
}
}
else{
Console.WriteLine("You must enter a positive number.");
}
}
catch(FormatException e){
Console.WriteLine("You must enter a whole number.");
}
}
}
}

Adrian Satmarel
28,633 PointsThank you. Yes, now I see we don't need the loop. We used it in previous videos in this tutorial, that's why I used it. I had some compiller error saying that the value shouldn't be null, so I added one more if. I have an additional question: what is that 'e' after the Exception in catch?
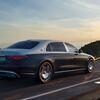
Balazs Peak
46,160 PointsThat could be anything, it's a variable name. I've named the exception (of type FormatException) that is passed to the "catch" scope with the variable name "e".
In this case, it's not even used in the scope. It could be, though, for example, I could write out the exception's message with the WriteLine. If I want to do that, I can use the "Message" property of the exception object.
Something like this:
catch(FormatException e){
Console.WriteLine("You must enter a whole number.");
Console.WriteLine(e.Message);
}
As you probably already realised, I can use any variable name, instead of "e". For example:
catch(FormatException myFavoriteExceptionNameForAdrian){
Console.WriteLine("You must enter a whole number.");
Console.WriteLine(myFavoriteExceptionNameForAdrian.Message);
}