Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial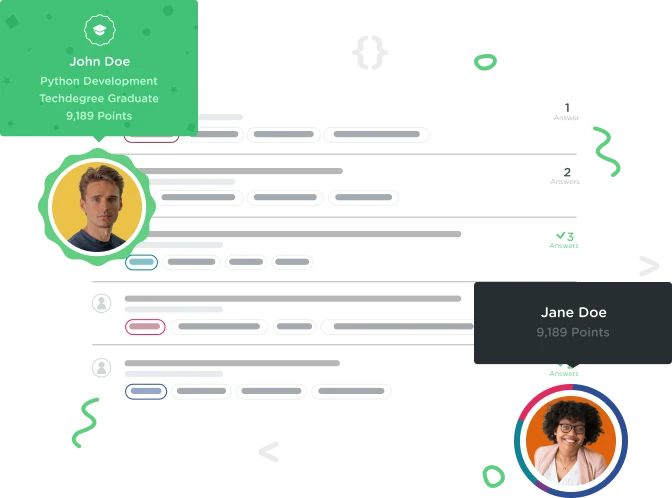

Joel Pendleton
19,230 PointsWhy isn't my code working?
var outputDiv = document.getElementById('output');
var students = [
{
name: 'Joel',
track: 'iOS',
achievements: 20,
points: 10000
},
{
name: 'Joe',
track: 'Swift',
achievements: 30,
points: 10430
},
{
name: 'Sam',
track: 'Design',
achievements: 10,
points: 1000
},
{
name: 'Tom',
track: 'Game development',
achievements: 23,
points: 43434
},
{
name: 'Peter',
track: 'Java',
achievements: 230,
points: 30000
},
];
function print(message) {
outputDiv.innerHTML = message;
}
function capitalize(s) {
// returns the first letter capitalized + the string from index 1 and out aka. the rest of the string
return s[0].toUpperCase() + s.substr(1);
}
var search = prompt('Who would you like to search for? Type quit to stop searching.');
function searchStudents (search) {
for (var i = 0; i < students.length; i += 1) {
function print(message) {
outputDiv.innerHTML = message;
}
for (var property in students[i]) {
if (search === students[i].name) {
if (students[i][property] != students[i]['name']) {
print('<p> ' + capitalize(property) + ': ' + students[i][property] + ' </p>');
} else {
print("<h2>" + capitalize(property) + ": " + students[i][property] + "</h2>");
}
} else if (search === 'quit') {
break;
}
}
}
if (outputDiv.hasChildNodes()) {
print('<h2 class = "no-results"> Please try searching again... </h2>');
}
}
searchStudents(search);
I want to use the print function I have defined to output results from this search program but for some reason it doesn't seem to work the only way I can get it to work is by using document.write but I want to be able to specify where to put the content - not just in the body.
If someone could help me that would be great :)
1 Answer
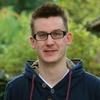
Martijn Schuijers
9,961 PointsHi Joel,
Your code doesn't work because the if-statement at the end of the searchStudents function is wrong. If there are results, the outputDiv will have child nodes (because you add them using your print function). Therefore it should be changed to:
if (!outputDiv.hasChildNodes()) {
print('<h2 class = "no-results"> Please try searching again... </h2>');
}
Another thing I noticed is that the print function replaces the inner HTML of the outputDiv. Therefore you will only see the number of points for a student instead of all properties. Instead of calling the print function multiple times it's better to construct the message first (by concatenating html strings) and then printing it:
function searchStudents (search) {
var message = '';
for (var i = 0; i < students.length; i += 1) {
for (var property in students[i]) {
if (search === students[i].name) {
if (students[i][property] != students[i]['name']) {
message += '<p> ' + capitalize(property) + ': ' + students[i][property] + ' </p>';
} else {
message += "<h2>" + capitalize(property) + ": " + students[i][property] + "</h2>";
}
} else if (search === 'quit') {
break;
}
}
}
print(message);
if (!outputDiv.hasChildNodes()) {
print('<h2 class = "no-results"> Please try searching again... </h2>');
}
}
Joel Pendleton
19,230 PointsJoel Pendleton
19,230 PointsThanks for your help!