Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial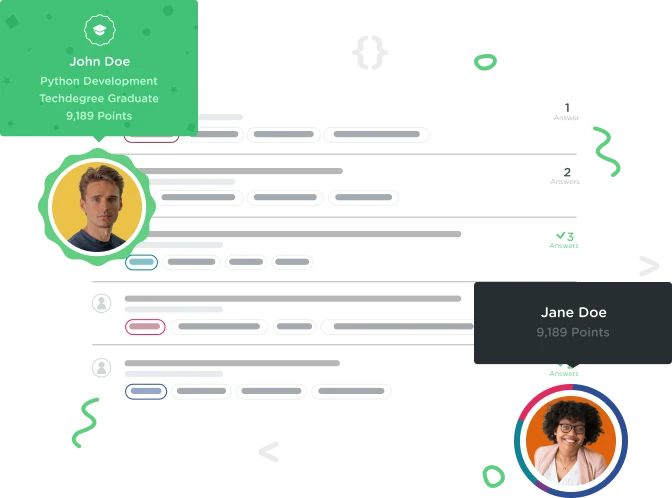
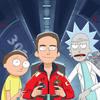
Andrew Chang
2,618 PointsWhy isn't my code working? It keeps showing "enter a letter: "
Hangman.java
public class Hangman{
public static void main(String[] args){
Game game = new Game("treehouse");
Prompter prompter = new Prompter(game);
while (game.getRemainingTries() > 0 && !game.isWon()){
prompter.promptForGuess();
prompter.displayProgress();
}
prompter.displayOutcome();
}
}
Game.java
class Game{
public static final int MAX_MISSES = 7;
private String answer;
private String hits;
private String misses;
public String getAnswer(){
return answer;
}
public Game(String answer){
this.answer = answer.toLowerCase();
hits = "";
misses = "";
}
private char normalizeGuess(char letter){
if(! Character.isLetter(letter)){
throw new IllegalArgumentException("A letter is required");
}
letter = Character.toLowerCase(letter);
if(misses.indexOf(letter) != -1 || hits.indexOf(letter) != -1){
throw new IllegalArgumentException(letter+"has already been guesses");
}
return letter;
}
public boolean applyGuess(String letters){
if(letters.length() == 0){
throw new IllegalArgumentException("No letter found");
}
return applyGuess(letters.charAt(0));
}
public boolean applyGuess(char letter){
letter = normalizeGuess(letter);
boolean isHit = answer.indexOf(letter) != -1;
if (isHit){
hits += letter;
} else{
misses += letter;
}
return isHit;
}
public int getRemainingTries() {
return MAX_MISSES - misses.length();
}
public String getCurrentProgress() {
String progress = "";
for(char letter : answer.toCharArray()) {
char display = '-';
if(hits.indexOf(letter) != -1){
display = letter;
}
progress += display;
}
return progress;
}
public boolean isWon(){
return getCurrentProgress().indexOf('-') == -1;
}
}
prompter.java
import java.util.Scanner;
class Prompter{
private Game game;
public Prompter(Game game){
this.game = game;
}
public boolean promptForGuess(){
Scanner scanner = new Scanner(System.in);
boolean isHit = false;
boolean isAcceptable = false;
do{
System.out.print("Enter a letter: ");
String guessInput = scanner.nextLine();
try{
isHit = game.applyGuess(guessInput);
}catch(IllegalArgumentException iae){
System.out.printf("%s. Please try again. %n",iae.getMessage());
}
}while(! isAcceptable);
return isHit;
}
public void displayProgress(){
System.out.printf("You have %d tries left to solve: %s%n",
game.getRemainingTries(),
game.getCurrentProgress());
}
public void displayOutcome(){
if(game.isWon()){
System.out.printf("You won with %d tries remaining!%n",game.getRemainingTries());
}else{
System.out.printf("Bummer! The word was %s.%n",game.getAnswer());
}
}
}
1 Answer
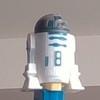
Teacher Russell
16,873 PointsThe problem is in the Prompter class. We're missing a line of code in the try/catch block. Take a look. The Prompter class needs to know that the guess is acceptable.