Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial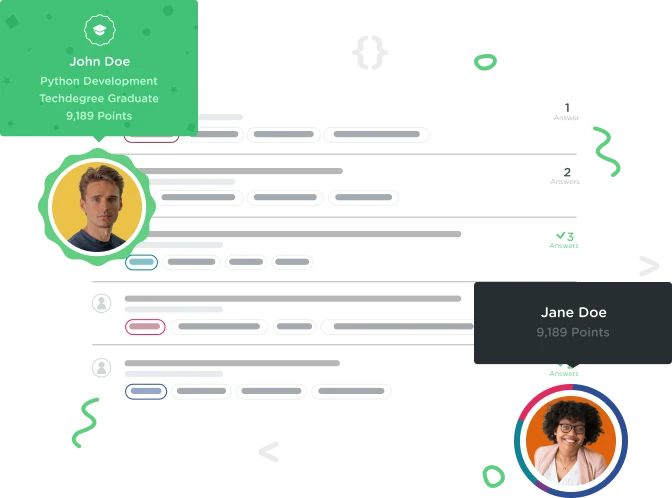
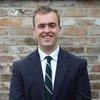
Edward Sapp
8,479 PointsWhy isn't my tuple switch working? It works in Xcode!
func fizzBuzz(n: Int) -> String { // Enter your code between the two comment markers switch (n % 3, n % 5){ case (0, ): return "Fizz" case(, 0): return "Buzz" case(0, 0): return "FizzBuzz" default: break } // End code return "(n)" }
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
switch (n % 3, n % 5){
case (0, _):
return "Fizz"
case(_, 0):
return "Buzz"
case(0, 0):
return "FizzBuzz"
default:
break
}
// End code
return "\(n)"
}
1 Answer
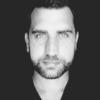
Jhoan Arango
14,575 PointsHello :
The way you have it, won't reach the "FizzBuzz" case, so if you put it on top, it will evaluate all possible conditions better..
For example if you pass it the number 15, since it could be a number that will trigger "fizz" or "buzz" conditions, then it won't reach all the way to the last case. So, it's best if you put the "FizzBuzz" case as the first condition.
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
switch (n % 3, n % 5){
case(0, 0):
return "FizzBuzz"
case (0, _):
return "Fizz"
case(_, 0):
return "Buzz"
default:
break
}
// End code
return "\(n)"
}
Hope it goes well, good luck.
Edward Sapp
8,479 PointsEdward Sapp
8,479 PointsThanks Jhoan. My biggest question is, what does "case (0, _)" mean? The way I read it, is something akin to:
"The case in which n%3==0 and n%5!=0"
In this case, it wouldn't matter whether the FizzBuzz condition was at the top or bottom. Thus, I must be reading something incorrectly, probably the _ symbol. What does the _ symbol represent in this case?
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsA tuple holds 2 values or more, if you want to ignore a specific one, you use the _ . So in this case it's evaluating one, but not the other.
Since you are switching on the tuple, it considers both values, unless you ignore one or the other.
Take a look at this example:
It will print this is a color, because the value that I passed in the case "Car" is not the same as " Ford ".
Good luck