Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial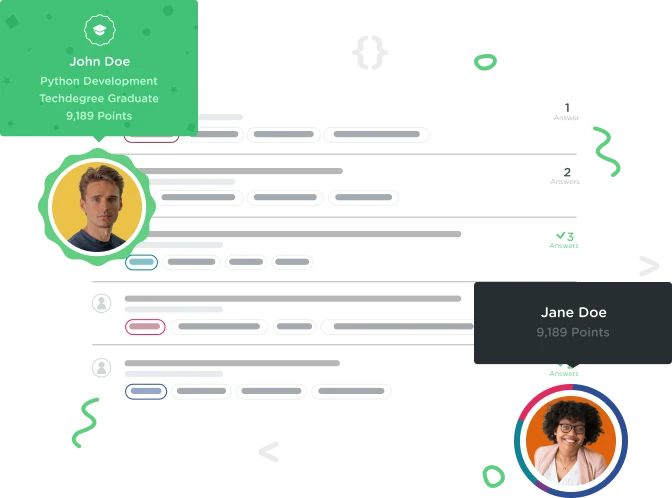
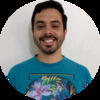
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsWhy isn't this code passing the Hand challenge?
I tried this code in Pycharm and it pretty much worked, except that when I try Hand.roll(2) I keep getting AttributeError: type object 'Hand' has no attribute 'roll'
Can anyone tell me if making small changes to this code it will work? I have seen other people code to pass this challenge, but I thought that my code should pass it too.
Thanks!
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self):
super().__init__(sides = 20)
from dice import D20
class Hand(list):
def __init__(self, size=0, die_class=None):
super().__init__() # I know I could check if die_class is truthy before this line, but I think the code should run fine for the purposes of the challenge.
for _ in range(size):
self.append(die_class())
@classmethod
def roll(cls, size, die_class = D20):
return cls(size, die_class)
@property
def total(self):
return sum(self)
1 Answer
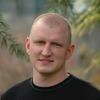
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsHey Ewerton Luna
I copy/pasted your snippets into the challenge and they seem to pass both tasks for me.
I would try clicking the Restart button on the challenge and then paste your code back into each .py
file. I initially suspected that you might a mixture of tab and space characters in your snippet. I checked for that first and your snippets seem to only be using space characters for indentation.
Give it another go, and let us know if it still doesn't pass. Also be sure you do not have any leading whitespace on the first line.
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsEwerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsHi, @Chris! Thank you very much! I tried the code again as you said and it worked! Thank you very much!!