Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial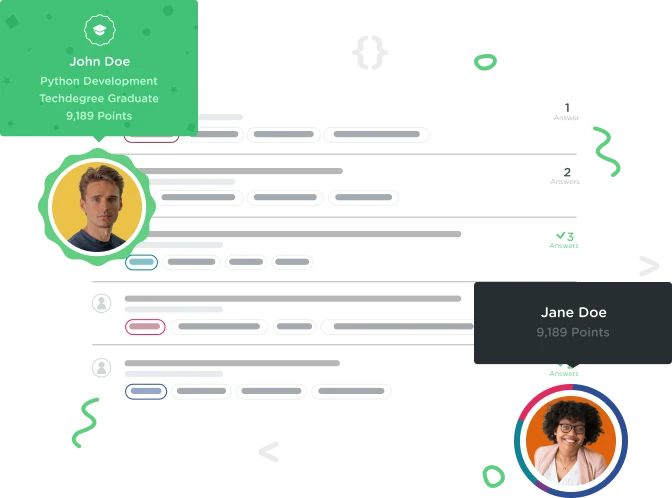

Ryan Scharfer
Courses Plus Student 12,537 PointsWhy isn't this code working?
I am simply trying to include jquery into my code, but this doesnt work in my Workspace.
<!DOCTYPE html>
<html>
<head>
<title>Take Evasive Action</title>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
<script src="http://code.jquery.com/jquery-1.11.0.min.js"></script>
</head>
<body>
<p class="warning">
<span>It's A Trap!</span>
</p>
<div class="image">
<img src="img/ackbar.gif" />
</div>
</body>
</html>
$(document).ready(myCode);
var myCode = function () {
$(".warning").hide().show("slow");
}
2 Answers

Michael Collins
433 PointsFirst, swap out the order of your scripts. I'm assuming that js/app.js is jQuery code that needs jQuery-1.11.0.min.js to run? So do this instead
<!DOCTYPE html>
<html>
<head>
<title>Take Evasive Action</title>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
<script src="http://code.jquery.com/jquery-1.11.0.min.js"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</head>
<body>
<p class="warning">
<span>It's A Trap!</span>
</p>
<div class="image">
<img src="img/ackbar.gif" />
</div>
</body>
</html>
Next, Function Declarations may be defined anywhere, but function expressions must be defined BEFORE they are called. So, this could work
var myCode = function () {
$(".warning").hide().show("slow");
}
$(document).ready(myCode);
However, if you want to defined the function AFTER document ready, you have to use a FUNCTION DECLARATION, like this
$(document).ready(myCode);
function myCode() {
$(".warning").hide().show("slow");
}

Robert Ho
Courses Plus Student 11,383 Pointshey Ryan,
try this:
$(document).ready(function(){
myCode();
});
var myCode = function () {
$(".warning").hide().show("slow");
}
I also just noticed that the order of your javascript links might be in the wrong order.
Switch them from this:
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
<script src="http://code.jquery.com/jquery-1.11.0.min.js"></script>
To this:
<script src="http://code.jquery.com/jquery-1.11.0.min.js"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
the jQuery has to be loaded before you can use its methods in your own code, or else they simply wouldn't exist if you are calling them in your own code before its even loaded.

Ryan Scharfer
Courses Plus Student 12,537 PointsThanks Robert! Your solution worked, but the next solution was a little easier, so I had to give him the "best answer". : )