Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial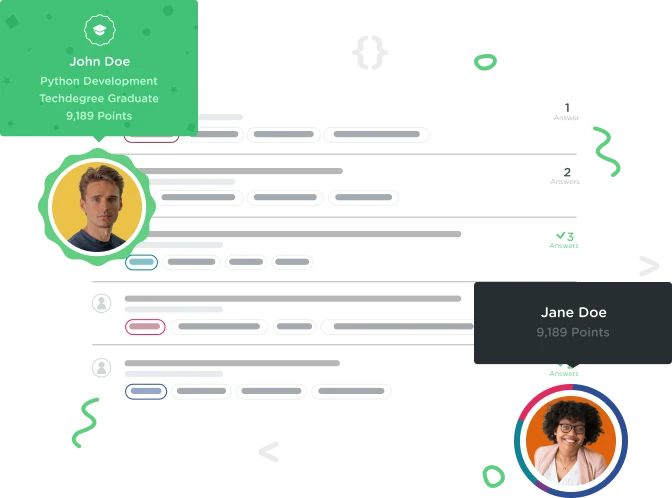
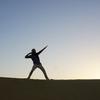
Alex G.
10,329 PointsWhy isn't this right?
I basically copied what was done in the lesson videos exactly.
class Student():
name = "Your Name"
def __init__(self, name, **kwargs):
self.name = name
for key, value in kwargs.items():
setattr(self, key, value)
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
return self.praise()
return self.reassurance()
1 Answer

andren
28,558 PointsThe init
method is fine but you have added a pair of parenthesis to the class declaration on the first line of code which does not belong there. If you remove them like this:
class Student: # Class declarations do not use parenthesis
name = "Your Name"
def __init__(self, name, **kwargs):
self.name = name
for key, value in kwargs.items():
setattr(self, key, value)
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
return self.praise()
return self.reassurance()
Then your code will work.
Alex G.
10,329 PointsAlex G.
10,329 PointsThat actually was not the error. The error turned out to be some indenting bug from when I copied + pasted from Sublime Text.
andren
28,558 Pointsandren
28,558 PointsI based the code in my answer on the code that is attached to your post, which is indented properly. So if you had an issue with your indentation then that must have occurred after posting this question.
The only issue with the code you posted is the one I pointed out, I verified that before I posted my answer.
Alex G.
10,329 PointsAlex G.
10,329 PointsThe code in my post was imported directly from my answer in the Code Challenge which was not working because some of my indents were spaces and some were tabs. I've had this issue before it must be a bug.
Removing the parenthesis made no difference. It is simply a convention to not use parenthesis unless you are using inheritance in which case it is necessary.
Thanks though.
andren
28,558 Pointsandren
28,558 PointsAfter doing a bit more research I realize you are correct about empty parenthesis being allowed so I'll concede that I was wrong on that point, Python is not my strongest language. Though that said the code attached to your post is in fact properly indented. If you copy it and paste it into the exercise you will be able to complete it without issue.
The reason why I wrongly pointed at the parenthesis was because it was literally the only non-standard thing I noticed about your code since the indentation problem is not present in the code in your post.
Anyway I'm glad you resolved your issue, even though my answer didn't end up being very useful, which I apologize for.
Edit: Changed some of my wording.