Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial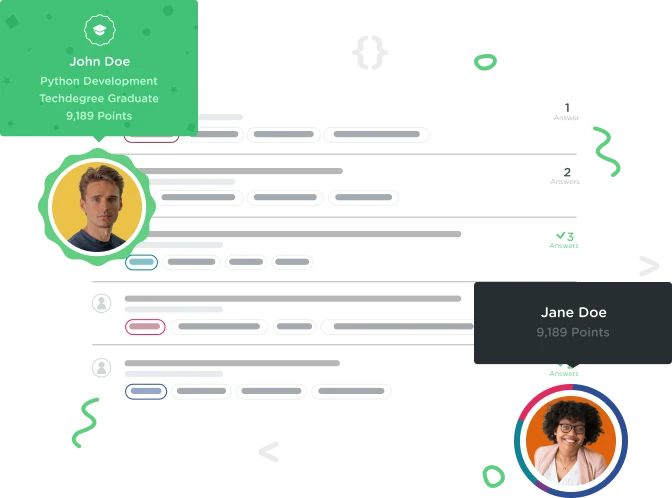
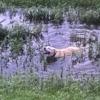
Kyle Vassella
9,033 PointsWhy isn't var guess defined at the top of the program?
The proper way that Dave shows to write this code is something like:
var upper = 10000
var randomNumber = getRandomNumber(upper)
var guess;
var attempts = 0
function getRandomNumber(upper) {
return Math.floor( Math.random() * upper ) + 1;
}
while (guess !== randomNumber) {
guess = getRandomNumber(upper)
attempts += 1;
}
alert("The random number was " + randomNumber + " and there were " + attempts + " attempts before a match!");
After looking at my completed program, I wondered why var guess was defined within the While loop and not at the top of the program with the other variables. So I tried moving var guess to the top of the page, like so:
var upper = 10000
var randomNumber = getRandomNumber(upper)
var guess = getRandomNumber(upper)
var attempts = 0
function getRandomNumber(upper) {
return Math.floor( Math.random() * upper ) + 1;
}
while (guess !== randomNumber) {
attempts += 1;
}
alert("The random number was " + randomNumber + " and there were " + attempts + " attempts before a match!");
This proved to be disastrous and caused the browser to freeze up each time I tried to preview it. Can someone explain to me why defining var guess at the top of the program results in such devastation? Why must it be defined within the While loop? I thought that when while(guess!==randomNumber) runs without having guess already defined, it might return an error. But instead the opposite was true - defining it first actually causes problems.
6 Answers
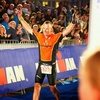
Steve Hunter
57,712 PointsHi Kyle,
If the value of guess
never changes because you only set it once when your program first starts, unless you are very lucky and guess
equals randomNumber
then the while
loop will just run forever.
Having guess = getRandomNumber(upper)
inside the while
loop means that the value of guess
changes at each iteration of the loop. That way, the loop will terminate once the correct guess is made.
Make sense?
Steve.
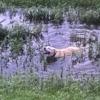
Kyle Vassella
9,033 PointsIt's funny how writing out the problem to ask a question can sometimes turn on a light bulb. Is the reason that guess can't be defined at the beginning because then each var would only run one time? Then var guess and var randomNumber would only generate one number each which in the vast majority of cases would result in two unequal numbers - thus causing the while loop to run indefinitely? Thus causing the freeze?
Wow, it's really cool that writing out the problem kind of helped me figure it out...I think. Am I a genius now?
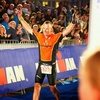
Steve Hunter
57,712 PointsYes, you are!
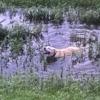
Kyle Vassella
9,033 PointsTo Dave's credit, he goes on to explain this exact phenomenon later in the video - apparently they are called endless loops and are really bad mmmkay. I had the video paused at 8 minutes, just before he explained it.
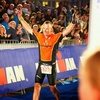
Steve Hunter
57,712 PointsEndless loops are bad, yes. If you create a loop with nothing inside the loop to change the condition you are testing, you'll be there a while.
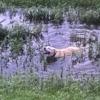
Kyle Vassella
9,033 PointsHaha, you beat me to it by a few seconds Steve, that was quick. Glad to have an answer, thanks for the lightning speed!
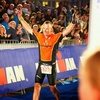
Steve Hunter
57,712 PointsI aim to please ...

izqcabvvli
4,111 PointsKyle, the variable should be initiated in the while loop because if it is defined before initiating the function the computer doesn't know what you are referring to. This caused the error.

Kevin Baxter
5,599 PointsThis is doesn't answer the question of how does the world guess get a value of a number...I don't understand how var guess = a number??? that doesn't make sense at all
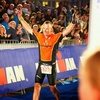
Steve Hunter
57,712 PointsHi Kevin,
We create four variables. upper
, randomNumber
, guess
and attempts
. We give upper
the value of 1,000 straight away. We store the returned value from getRandomNumber()
inside randomNumber
. We initialize attempts
to zero because we haven't made any attempts yet.
We then define the getRandomNumber()
function which just returns a random value between zero and upper
. So, it'll be between nought and one thousand.
Next, we create a while
loop which will continue looping while
the value within guess
(which is nothing at the moment - maybe we should have initialised it to a number greater than upper
? Semantics) does not equal the value held in randomNumber
. The first thing the loop does is generate another random number and store that within guess
. We then increment the number inside attempts
. The value of guess
is once again compared to the value within randomNumber
and the code will loop again if they are not equal. A new random number will be assigned to guess
and attempts
will be incremented again. And on we go until the condition of the test stops the loop.
I hope that helps.
Steve.

Rafael Ramirez-Gaston
3,898 Pointsvariable declarations can go on top of the program, but you can't define an undeclared variable
Matthias Nörr
8,614 PointsMatthias Nörr
8,614 PointsWhy does the computer guess a number anyway? Why don't I have to tell him first, what the variable "guess" is supposed to do?