Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial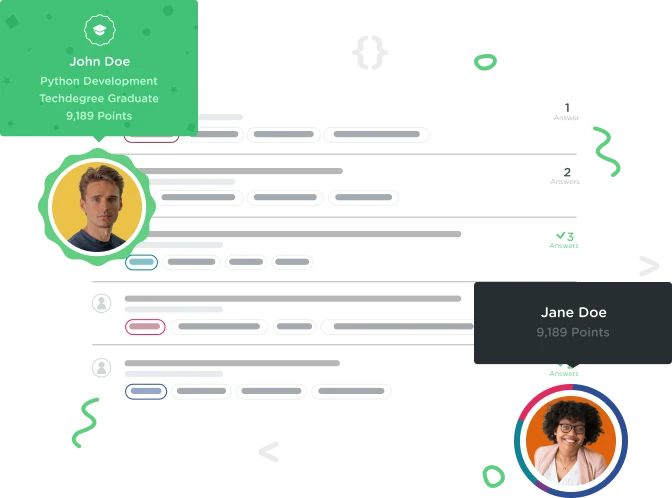
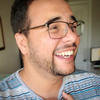
brevans26
15,198 PointsWhy it asks for two numbers but it does not accept integers?
Why this problem asks you to "create a new function named max which accepts TWO NUMBERS as arguments (you can name the arguments, WHATEVER YOU LIKE)." and it does not accept integers?
For example: I was trying
function max (13, 24) {
if (24 > 13) {
return 24
} else {
return 13
}
}
Although the way of thinking is correct, why it does not go through and when I try replacing the numbers by letters, I get it?
function max (a, b) {
if (a> b) {
return a
} else {
return b
}
}
1 Answer

andren
28,558 PointsBecause parameters (which is what you define in the parenthesis of the method declaration) are essentially variables that gets assigned a value when the function is called. Having a literal value like a string or number as a parameter is invalid (it's literally not allowed by JavaScript) as you cannot assign a new value to a string or number.
The task specifies it will pass two numbers as arguments, meaning that the two parameters you define will be set equal to numbers when the function is called. For example:
function max (a, b) { // a and b are parameters
// a will contain 3
// b will contain 5
if (a > b) {
return a
} else {
return b
}
}
max(3, 5) // 3 and 5 are arguments
Arguments and parameters are linked, but they are not the same thing. When you pass an argument into a function it gets assigned to the parameter that matches its position. In other words the first argument (3) is assigned to the first parameter (a) and the second argument (5) is assigned to the second parameter (b).
The entire point of parameters is that they allow the function to take in values from outside the function as it is called. Which allows it to generate different results based on what was passed into it. Therefore even if it was valid to use literal values as parameters it would be entirely pointless, as the function would work exactly the same as if it was declared without any parameters.