Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial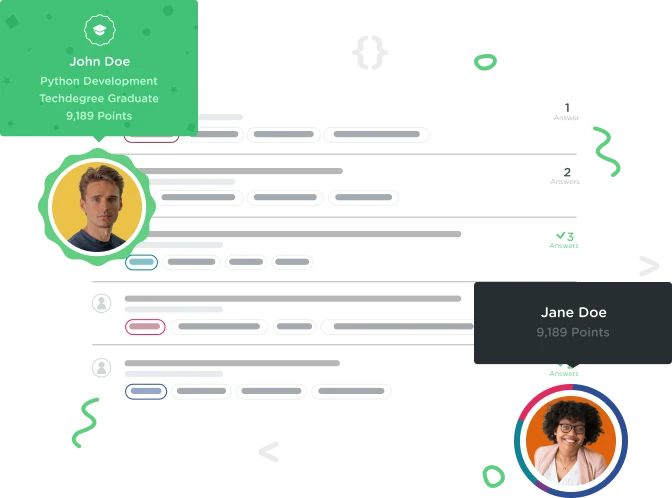

Jiho Song
16,469 PointsWhy it is wrong? Java I
where to fix????
public class Forum {
private String topic;
// TODO: add a constructor that accepts a topic and sets the private field topic
private Forum(String topic)
{
this.topic=topic;
}
public String getTopic() {
return topic;
}
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
}
public class User {
// TODO: add private fields for firstName and lastName
private String firstName;
private String lastName;
private User(String firstName, String lastName) {
// TODO: set and add the private fields
this.firstName=firstName;
this.lastName=lastName;
}
public String getFirstName(){
return firstName;
}
public String getLastName(){
return lastName;
}
// TODO: add getters for firstName and lastName
}
public class ForumPost {
private User author;
private String title;
private String description;
private ForumPost(User author, String title, String description){
this.author = author;
this.title = title;
this.description = description;
}
// TODO: add a constructor that accepts the author, title and description
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
User author = new User("Jiho", "Song");
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost(author, "HowtoJava", "easy");
forum.addPost(post);
}
}
2 Answers
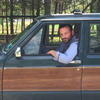
Alexandre Babeanu
10,947 PointsHello,
So first of all your constructors need to be public so they can be accessed in the Main class.
change private Forum, private User, and private ForumPost to public Forum public User and public ForumPost.
then when fixing the main.java :
you need to create a new User object with the first name and last name contained in the args array as parameters for the User constructor. NOT Jiho Song
change "Jiho","Song" to args[0], args[1]

Jiho Song
16,469 PointsThanks for teaching but what ensure that specifically name is going to be saved in argc[0], argc[1]? It could be argc[3] or even others?
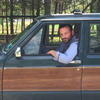
Alexandre Babeanu
10,947 PointsSorry Jiho, I don't know. why don't you ask team tree house? Maybe it is related to the lesson. Good luck!

Jiho Song
16,469 PointsHowever where does this program takes value from the user? It should input the info from user by somewhat method such as console.readLine, however i cant not find any. I sort of understand it not 100%
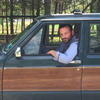
Alexandre Babeanu
10,947 Pointstaking value from the user is not in the scope of that prograam. It could be done in many various ways. You have to assume some external program retreives info from the user and formats it as an array. The array is then passed as a parameter to main(). Jiho this is just an exercise on constructors its not meant as a fully working prgram.
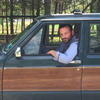
Alexandre Babeanu
10,947 PointsIf you found my response helpful please vote it up.
Alexandre Babeanu
10,947 PointsAlexandre Babeanu
10,947 Pointswhat is the thrown error?