Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial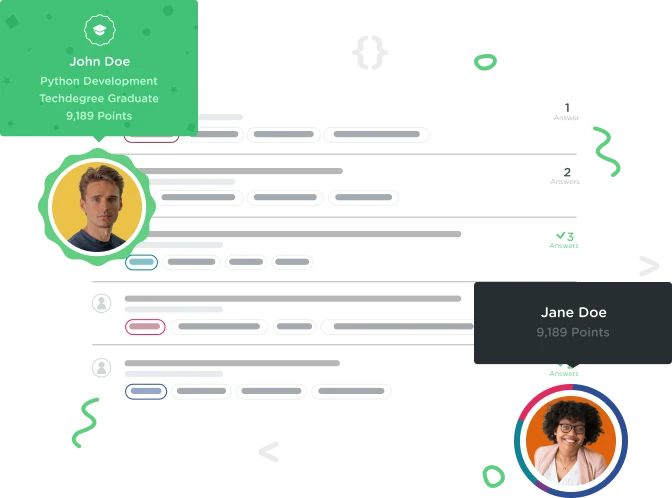
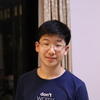
Thanitsak Leuangsupornpong
7,490 PointsWhy it keep saying can't import name "taco".
How to import it thanks!
import unittest
from playhouse.test_utils import test_database
from peewee import *
import tacocat
from models import User, Taco
TEST_DB = SqliteDatabase(':memory:')
TEST_DB.connect()
TEST_DB.create_tables([User, Taco], safe=True)
USER_DATA = {
'email': 'test_0@example.com',
'password': 'password'
}
class UserModelTestCase(unittest.TestCase):
@staticmethod
def create_users(count=2):
for i in range(count):
User.create_user(
email='test_{}@example.com'.format(i),
password='password'
)
def test_create_user(self):
with test_database(TEST_DB, (User,)):
self.create_users()
self.assertEqual(User.select().count(), 2)
self.assertNotEqual(
User.select().get().password,
'password'
)
def test_create_duplicate_user(self):
with test_database(TEST_DB, (User,)):
self.create_users()
with self.assertRaises(ValueError):
User.create_user(
email='test_1@example.com',
password='password'
)
class TacoModelTestCase(unittest.TestCase):
def test_taco_creation(self):
with test_database(TEST_DB, (User, Taco)):
UserModelTestCase.create_users()
user = User.select().get()
Taco.create(
user=user,
protein='chicken',
shell='flour',
cheese=False,
extras='Gimme some guac.'
)
taco = Taco.select().get()
self.assertEqual(
Taco.select().count(),
1
)
self.assertEqual(taco.user, user)
class ViewTestCase(unittest.TestCase):
def setUp(self):
tacocat.app.config['TESTING'] = True
tacocat.app.config['WTF_CSRF_ENABLED'] = False
self.app = tacocat.app.test_client()
class UserViewsTestCase(ViewTestCase):
def test_registration(self):
data = {
'email': 'test@example.com',
'password': 'password',
'password2': 'password'
}
with test_database(TEST_DB, (User,)):
rv = self.app.post(
'/register',
data=data)
self.assertEqual(rv.status_code, 302)
self.assertEqual(rv.location, 'http://localhost/')
def test_good_login(self):
with test_database(TEST_DB, (User,)):
UserModelTestCase.create_users(1)
rv = self.app.post('/login', data=USER_DATA)
self.assertEqual(rv.status_code, 302)
self.assertEqual(rv.location, 'http://localhost/')
def test_bad_login(self):
with test_database(TEST_DB, (User,)):
rv = self.app.post('/login', data=USER_DATA)
self.assertEqual(rv.status_code, 200)
def test_logout(self):
with test_database(TEST_DB, (User,)):
# Create and login the user
UserModelTestCase.create_users(1)
self.app.post('/login', data=USER_DATA)
rv = self.app.get('/logout')
self.assertEqual(rv.status_code, 302)
self.assertEqual(rv.location, 'http://localhost/')
def test_logged_out_menu(self):
rv = self.app.get('/')
self.assertIn("sign up", rv.get_data(as_text=True).lower())
self.assertIn("log in", rv.get_data(as_text=True).lower())
def test_logged_in_menu(self):
with test_database(TEST_DB, (User,)):
UserModelTestCase.create_users(1)
self.app.post('/login', data=USER_DATA)
rv = self.app.get('/')
self.assertIn("add a new taco", rv.get_data(as_text=True).lower())
self.assertIn("log out", rv.get_data(as_text=True).lower())
class TacoViewsTestCase(ViewTestCase):
def test_empty_db(self):
with test_database(TEST_DB, (Taco,)):
rv = self.app.get('/')
self.assertIn("no tacos yet", rv.get_data(as_text=True).lower())
def test_taco_create(self):
taco_data = {
'protein': 'chicken',
'shell': 'flour',
'cheese': False,
'extras': 'Gimme some guac.'
}
with test_database(TEST_DB, (User, Taco)):
UserModelTestCase.create_users(1)
self.app.post('/login', data=USER_DATA)
taco_data['user'] = User.select().get()
rv = self.app.post('/taco', data=taco_data)
self.assertEqual(rv.status_code, 302)
self.assertEqual(rv.location, 'http://localhost/')
self.assertEqual(Taco.select().count(), 1)
def test_taco_list(self):
taco_data = {
'protein': 'chicken',
'shell': 'flour',
'cheese': False,
'extras': 'Gimme some guac.'
}
with test_database(TEST_DB, (User, Taco)):
UserModelTestCase.create_users(1)
taco_data['user'] = User.select().get()
Taco.create(**taco_data)
rv = self.app.get('/')
self.assertNotIn('no tacos yet', rv.get_data(as_text=True))
self.assertIn(taco_data['extras'], rv.get_data(as_text=True))
if __name__ == '__main__':
models.initialize()
try:
models.User.create_user(
email='tacolover@taco.com',
password='password'
)
except ValueError:
pass
import datetime
from flask.ext.bcrypt import generate_password_hash
from flask.ext.login import UserMixin
from peewee import *
DATABASE = SqliteDatabase('Our Moment.db')
class User(UserMixin, Model):
username = CharField(unique=True)
email = CharField(unique=True)
password = CharField(max_length=100)
joined_at = DateTimeField(default=datetime.datetime.now)
is_admin = BooleanField(default=False)
class Meta:
database = DATABASE
order_by = ('-joined_at',)
def get_posts(self):
return Post.select().where(Post.user == self)
def get_stream(self):
return Post.select().where(
(Post.user << self.following()) |
(Post.user == self)
)
def following(self):
"""The user that we are following."""
return(
User.select().join(
Relationship, on=Relationship.to_user
).where(
Relationship.from_user == self
)
)
def followers(self):
"""Get users following the current user"""
return(
User.select().join(
Relationship, on=Relationship.from_user
).where(
Relationship.to_user == self
)
)
@classmethod
def create_user(cls, username, email, password, admin=False):
try:
with DATABASE.transaction():
cls.create(
username=username,
email=email,
password=generate_password_hash(password),
is_admin=admin)
except IntegrityError:
raise ValueError("User already exists")
class Post(Model):
timestamp = DateTimeField(default=datetime.datetime.now)
user = ForeignKeyField(
rel_model=User,
related_name='posts'
)
content = TextField()
class Meta:
database = DATABASE
order_by = ('-timestamp',)
class Relationship(Model):
from_user = ForeignKeyField(User, related_name='relationships')
to_user = ForeignKeyField(User, related_name='related_to')
class Meta:
database = DATABASE
indexes = (
(('from_user', 'to_user'), True)
)
def initialize():
DATABASE.connect()
DATABASE.create_tables([User, Post, Relationship], safe=True)
DATABASE.close()
from flask_wtf import Form
from wtforms import StringField, PasswordField, TextAreaField
from wtforms.validators import (DataRequired, Regexp, ValidationError, Email,
Length, EqualTo)
from models import User
def name_exists(form, field):
if User.select().where(User.username == field.data).exists():
raise ValidationError('User with that name already exists.')
def email_exists(form, field):
if User.select().where(User.email == field.data).exists():
raise ValidationError('User with that email already exists.')
class RegisterForm(Form):
username = StringField(
'Full Name',
validators=[
DataRequired(),
Regexp(
r'^[a-zA-Z0-9_]+$',
message=("Full Name should be one word(no space), letters, "
"numbers, and underscores only.")
),
name_exists
])
email = StringField(
'Email',
validators=[
DataRequired(),
Email(),
email_exists
])
password = PasswordField(
'Password',
validators=[
DataRequired(),
Length(min=2),
EqualTo('password2', message='Passwords must match')
])
password2 = PasswordField(
'Confirm Password',
validators=[DataRequired()]
)
class LoginForm(Form):
email = StringField('Email', validators=[DataRequired(), Email()])
password = PasswordField('Password', validators=[DataRequired()])
class PostForm(Form):
content = TextAreaField("Share your moments to your friends!", validators=[DataRequired()])
{% extends 'layout.html' %}
{% block content %}
<h2>Tacos</h2>
{% if tacos.count() %}
<table class="u-full-width">
<thead>
<tr>
<th>Protein</th>
<th>Cheese?</th>
<th>Shell</th>
<th>Extras</th>
</tr>
</thead>
<tbody>
{% for taco in tacos %}
<!-- taco attributes here -->
{% endfor %}
</tbody>
</table>
{% else %}
<!-- message for missing tacos -->
{% endif %}
{% endblock %}
1 Answer
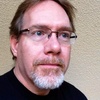
Chris Freeman
Treehouse Moderator 68,423 PointsYour import statement says: from models import User, Taco
. Looking at your models.py
file, I do not see a Taco
class to be imported. Where are you expecting to find Taco
?
Thanitsak Leuangsupornpong
7,490 PointsThanitsak Leuangsupornpong
7,490 PointsOk, I need to put the Taco class, with the pass in side right?
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsCorrect. You may also want to add some attributes in the same way it was done for the User class, like
username
etc.