Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial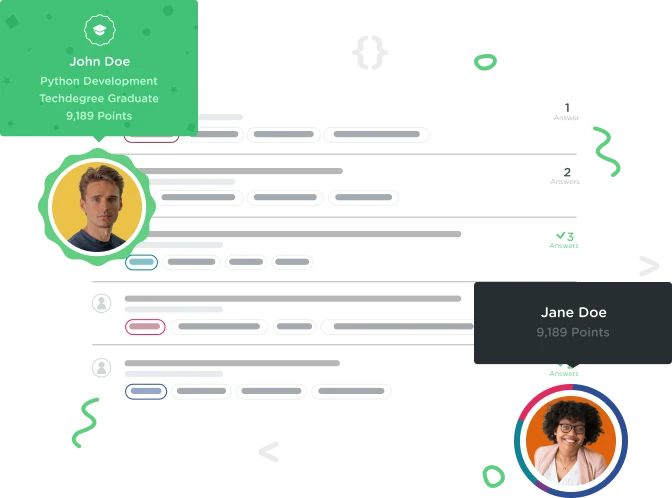
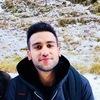
Anmol Bajaj
5,556 PointsWhy it shows : There was an syntax error in parse.
I don't know why parseInt is showing error !!
function max(no1, no2){
if (Number.parseInt(no1)>Number.parseInt(no2)){
return no1;
}else {
return no2;
}
2 Answers

andren
28,558 PointsYou are missing a closing brace } at the end of your code to close the function. Also since this function will be passed in numbers, not strings, you don't need to parse them. Like this:
function max(no1, no2) {
if (no1 > no2) { // No parsing needed
return no1;
} else {
return no2;
}
} // Added missing closing brace
It's also worth mentioning that Number.parseInt
is not supported in these challenges since it was introduced in JavaScript's ES6/ES2015 version which is only supported in some of the later challenges. The global parseInt
function (without Number
) is supported but as mentioned not needed for this challenge.
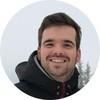
John Lack-Wilson
8,181 PointsNumber.parseInt() takes two parameters, whereas you have just supplied one. See here on the documentation

andren
28,558 PointsThe second parameter is optional, so that would actually not cause any issues by itself.
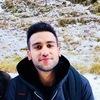
Anmol Bajaj
5,556 PointsYes andren is right, the second parameter is optional.
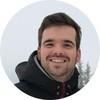
John Lack-Wilson
8,181 PointsI see, odd that the docs didn't specify optional, unless I mis-read

andren
28,558 PointsThe syntax example for the command is listed like this:
Number.parseInt(string,[ radix ])
Arguments enclosed in square brackets are optional, but it's true that it should have been specified next to the actual parameter description as well. Since MDN documentation is written by hand it is not always super consistent when it comes to things like this.
It's also possible it's not explicitly marked as optional because passing both arguments is the recommended way of using parseInt
, since that reduces chances of bugs occurring form the wrong number base being assumed. Though in actual practice it's rare to see both parameters used.
Anmol Bajaj
5,556 PointsAnmol Bajaj
5,556 PointsIt worked after I added a closing brace } at the end of the code. Otherwise, everything else was fine. Thanks for the help andren.