Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial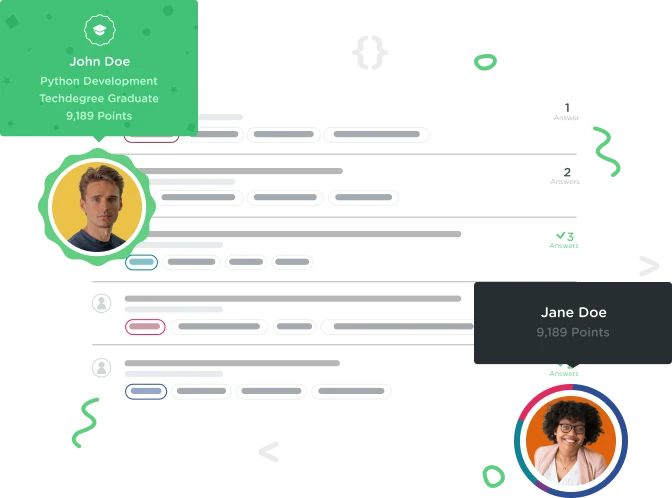

Reza Sorasti
491 PointsWhy Jquary is used here instead of just pure HTML and CSS?
Here is the index.html file:
code
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<div id="menu">
<ul>
<li class="selected"><a href="index.html">Home</a></li>
<li><a href="about.html">About</a></li>
<li><a href="contact.html">Contact</a></li>
<li><a href="support.html">Support</a></li>
<li><a href="faqs.html">FAQs</a></li>
<li><a href="events.html">Events</a></li>
</ul>
</div>
<h1>Home</h1>
<p>This is the home page.</p>
<script src="http://code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
Here is the about.html file:
code
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<div id="menu">
<ul>
<li><a href="index.html">Home</a></li>
<li class="selected"><a href="about.html">About</a></li>
<li><a href="contact.html">Contact</a></li>
<li><a href="support.html">Support</a></li>
<li><a href="faqs.html">FAQs</a></li>
<li><a href="events.html">Events</a></li>
</ul>
</div>
<h1>About</h1>
<p>This is the about page.</p>
<script src="http://code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
The rest of the html files are same except each of that pages are the selected ones.
Here is the style.css file:
code
* {
margin: 0;
padding: 0;
font-family: sans-serif;
}
body {
background: #fff;
}
#menu {
background: #384047;
height: 60px;
padding: 0 5px 0;
text-align: center;
}
ul {
list-style: none;
}
ul li {
display: inline-block;
width: 84px;
text-align: center;
}
ul li a {
color: #fff;
width: 100%;
line-height: 60px;
text-decoration: none;
}
ul li.selected {
background: #fff;
}
ul li.selected a {
color: #384047;
}
select {
width: 94%;
margin: 11px 0 11px 2%;
float: left;
}
h1 {
margin: 40px 0 10px;
text-align: center;
color: #384047;
}
p {
text-align: center;
color: #777;
}
/** Start Coding Here **/
/**
Modify CSS to hide links on small width and show button and select
Also hides select and button on larger width and show's links
**/
@media (min-width: 320px) and (max-width: 568px) {
#menu ul {
display:none;
}
}
@media (min-width: 568px) {
#menu select, #menu button {
display:none;
}
}
And here is the app.js file:
code
//Create a select and append to #menu
var $select = $("<select></select>");
$("#menu").append($select);
//Cycle over menu links
//for each of the anchor links the below function gets called
$("#menu a").each(function(){
var $anchor = $(this);
//Create an option
var $option = $("<option></option>");
//Deal with selected options depending on current page
if($anchor.parent().hasClass("selected")) {
$option.prop("selected", true);
}
//option's value is the href
$option.val($anchor.attr("href"));
//option's text is the text of link
$option.text($anchor.text());
//append option to select
$select.append($option);
});
//Create button
var $button = $("<button>Go</button>");
$("#menu").append($button);
//Now, we want to bind a click handler to that
//particular button.
$button.click(function(){
//we are getting the selected value of the
// select box and setting it to window's location
//alert($select.val());
window.location = $select.val();
});
//Bind change listener to the select
//$select.change(function(){
//Go to select's location
// window.location = $select.val();
//});
I don't fully understand why Jquary is used here.
1) Why are we creating a detached DOM objects like Button and Select?
2) Why could we just use the HTML to create the Button and Select Elements using the <button> and <select> tags?
3) Can somebody help me to understand why we are using Jquary here instead of just HTML and CSS? For some reason I don't fully see why there is a need to use Jquary.
4) I don't even see a need to use Javascript here either. It seems like the whole thing can be done by just using HTML and CSS.
2 Answers
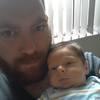
Daniel Bell
17,178 PointsThe navigation menu in the HTML is written in a very common/standard way, with a list of links. The way the jQuery code generates it is done for aesthetic look and feel and not because form elements are necceserily appropriate here. Our markup we should try to ensure is focussed purely on structuring our content. By using jQuery here, if you ever want to change the way the links look (say back to just a list of links) you just delete the javascript. If you wrote the options and select items into the HTML you'd need to go and remove them all again to change back.

Reza Sorasti
491 PointsI don't really understand the whole removing and deleting thing that you are mentioning here. May be if you want, you can try explaining it a different way. Why are we just not using HTML and CSS. Using Media Quary we can make the text of the links appear when the width of the browser gets big and disappear the text of the links when the the browser width gets small and again make appear the select element and the button element when the width of the browser gets small and dispear them when it get bigs. So, where in this whole project we do need to use Jquary? Where in this whole project are we manipulating a DOM? Even if we are manipulating the DOM, why are we not just using Javascript to do manipulate the DOM?