Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial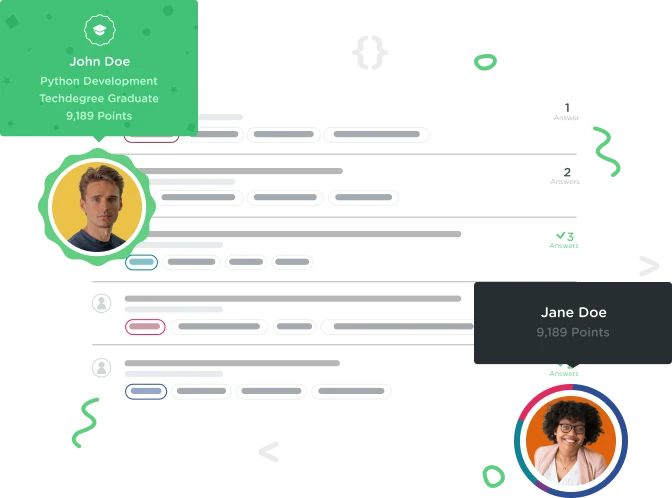

Dylan Carter
4,780 Pointswhy multiple load methods?
What is the benefit of having multiple load methods?
public void load() {
load(MAX_PEZ);
}
public void load(int pezAmount) {
mPezCount += pezAmount;
System.out.println("you loaded some pez!");
}
this is what we had from this lesson, im trying to understand why you would want to do this rather than just rename the top method fullyLoad or something like that to avoid confusion?
[MOD: added ```java markdown formatting -cf]
3 Answers

r h
68,552 PointsIt's called overloading a function, and it lets you reuse a method in multiple situations without having to remember two different function names.

Rulon Taylor
2,762 PointsThe no parameter method automatically loads the maximum number of Pez to the dispenser. This assumes that the dispenser is empty and the user wishes a complete fill.
The second load method provides flexibility by allowing the user to specify a number of Pez to load. This way, a few Pez can be added if the dispenser is not completely empty, or the dispenser can be partially filled instead of completely filled.
Overloading the load method in this way provides flexibility in how the method is used.

Drew Arrow Collins
Courses Plus Student 226 PointsI get it, I just don't understand what the MAX_PEZ is doing there.
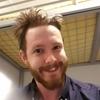
Chris Tvedt
3,795 PointsIm going to try to explain (sorry for opening old thread, but might help others if i get this right :) )
public void load() {
load(MAX_PEZ);
}
If you here call "dispenser.load()", this means "Fill the pez dispenser with "MAX_PEZ" (preset to 12 pez), assuming the dispenser is empty. But if it is not empty, lets say there are 3 pez left in the dispenser, then the "dispenser.load()" would be stopped because "!isEmpty". So...
That is where this part of the code comes in to the picture:
public void load(int pezAmount) {
mPezCount += pezAmount;
}
here you can specify the number of pez you want to put in the dispenser. Say 9 (since there are 3 in the dispenser already), by calling "dispenser.load(9)" this will increase the "mPezCount" by 9. Is this a good explanation of these fields? (These are fields right? or is it methods? Or functions? :P)
Hope this helps people understand the overloading part. Did i get this right Craig Dennis ?
Chris
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsChanged comment to Answer.
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsOther languages, such as Python, allow assigning a default value for a parameter if it is not passed as an argument.
Since Java doesn't have this capability, function overloading is the want to specify actions if no arguments are present.
Ismael Rac
Courses Plus Student 15,244 PointsIsmael Rac
Courses Plus Student 15,244 PointsThanks for Video Link very helfull