Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial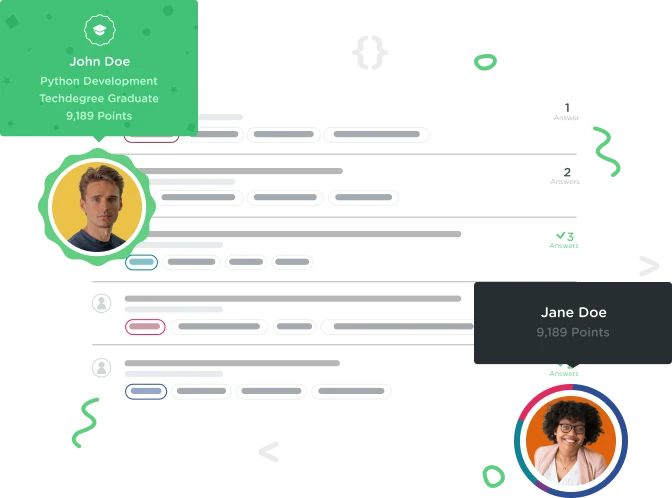

Liang Junwen
582 PointsWhy my code is wrong?
class Product(object):
_price = 0
tax_rate = 0.12
def __init__(self, base_price):
self._price = base_price
@property
def price(self):
return self._price + (self._price * self.tax_rate)
@price.setter
def price(self, value):
self._price = value / (1 + self.tax_rate)
The quiz is: We need to be able to set the price of a product through a property setter. Add a new setter (@price.setter) method to the Product class that updates the _price attribute.
But when I submit my answer, I just receive: Bummer: Didn't correctly set the @price
property.
Why?
class Product(object):
_price = 0
tax_rate = 0.12
def __init__(self, base_price):
self._price = base_price
@property
def price(self):
return self._price + (self._price * self.tax_rate)
@price.setter
def price(self, value):
self._price = value / (1 + self.tax_rate)
2 Answers
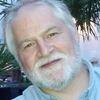
Jeff Muday
Treehouse Moderator 28,716 PointsIt looks like you know what you are doing, but the challenge is simple...
On this specific challenge, you are ONLY supposed to write a price setter. Nothing more.
@price.setter
def price(self, value):
self._price = value
Good luck with Python, it's a great skill and fun to program!
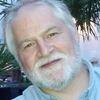
Jeff Muday
Treehouse Moderator 28,716 PointsI am not sure what is happening with that... did you save the Python file before your run? It is easy to screw up the setter because Python doesn't check that you named the setter as an exact match.
Here is a more "full featured example" of code. Feel free to use the style below for your own class. The thing I love about Python is this could be written in ones own personal style that might be more efficient or clear or better PEP-8 compliance.
class Product(object):
# we use TWO underscores to denote these are PRIVATE class values
__name = "object"
__price = 0
__tax_rate = 0
__discount = 0
def __init__(self, name, price=0, discount=0, tax_rate=0):
self.__name = name
self.__price = price
self.__discount = discount
self.__tax_rate = tax_rate
@property
def price(self):
return self.__price
@price.setter
def price(self, value):
self.__price = value
@property
def discount(self):
return self.__discount
@discount.setter
def discount(self, value):
self.__discount = value
@property
def tax_rate(self):
return self.__tax_rate
@tax_rate.setter
def tax_rate(self, value):
self.__tax_rate = value
def calculated_discount_price(self, taxable=False):
# callable method
item_price = self.__price - (self.__price * self.__discount)
if taxable:
tax = self.__tax_rate
else:
tax = 0
return item_price + (item_price * tax)
def calculated_retail_price(self, taxable=False):
# callable method
# full retail price is discounted price with a zero discount
item_price = self.__price
if taxable:
tax = self.__tax_rate
else:
tax = 0
return item_price + (item_price * tax)
@property
def retail_price(self):
return self.calculated_retail_price()
@property
def retail_price_tax(self):
return self.calculated_retail_price(taxable=True)
@property
def discount_price(self):
return self.calculated_discount_price()
@property
def discount_price_tax(self):
return self.calculated_discount_price(taxable=True)
def __str__(self):
return """
name: "{}"
price: ${}
discount: {} %
discount price: ${}
tax rate: {} %
--------------------
price with tax: ${}
price with tax and discount ${}
""".format(
self.__name,
self.__price,
self.__discount * 100,
self.discount_price,
self.__tax_rate * 100,
self.retail_price_tax,
self.discount_price_tax
)
if __name__ == '__main__':
basic_grocery_tax_rate = 0.07
luxury_item_tax_rate = 0.12
apple = Product("Granny Smith Apple")
apple.price = 1.25
apple.tax_rate = 0.08
apple.discount = 0.25
print(apple)
coke = Product("Coca Cola (six-pack)",
price=3.00,
discount=0.10,
tax_rate=luxury_item_tax_rate)
print(coke)
simple = Product("Simple Simon Snack Pie",
price=1.00,
discount=0.10,
tax_rate=luxury_item_tax_rate)
print(simple)
Here is the output
name: "Granny Smith Apple"
price: $1.25
discount: 25.0 %
discount price: $0.9375
tax rate: 8.0 %
--------------------
price with tax: $1.35
price with tax and discount $1.0125
name: "Coca Cola (six-pack)"
price: $3.0
discount: 10.0 %
discount price: $2.7
tax rate: 12.0 %
--------------------
price with tax: $3.36
price with tax and discount $3.024
name: "Simple Simon Snack Pie"
price: $1.0
discount: 10.0 %
discount price: $0.9
tax rate: 12.0 %
--------------------
price with tax: $1.12
price with tax and discount $1.008

Liang Junwen
582 PointsHi, Jeff! Thank you so much for your detailed code, really helpful~~
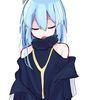
Kurobe Kuro^T_T^
5,369 PointsOHH MY LORD YOUR AMAZING
Liang Junwen
582 PointsLiang Junwen
582 PointsHi, Jeff. Thanks for your quickly reply. Your code is right, I really did not consider the challenge was such easy. But this challenge does not make sense for me. For example:
The output is 112, that means the value of attribute apple.price has been changed from what I assigned to which is 100 to 112. So what is the function of this class definition?