Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial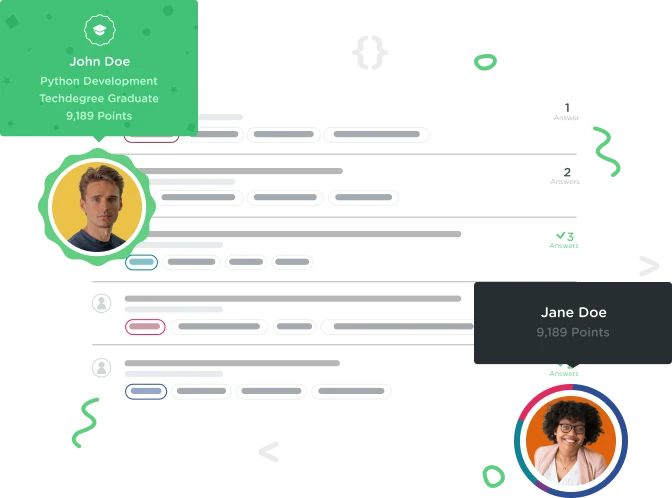
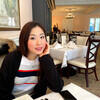
Hanwen Zhang
20,084 PointsWhy my code is wrong? I thought the paragraph is the child to the section.
Next, change the color of each child paragraph to blue.
(Remember: paragraphs is a collection of elements, so you'll first need to use a loop to access each element in the collection.)
var section = document.querySelector('section');
var paragraphs = section.children;
for ( let i=0; i<section.length, i++ ) {
section [i] .style.color= "blue';
}
<!DOCTYPE html>
<html>
<head>
<title>Child Traversal</title>
</head>
<body>
<section>
<p>This is the first paragraph</p>
<p>This is a slightly longer, second paragraph</p>
<p>Shorter, last paragraph</p>
</section>
<footer>
<p>© 2019</p>
</footer>
<script src="app.js"></script>
</body>
</html>
1 Answer
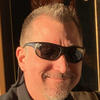
Peter Vann
36,427 PointsHi Hanwen!
Your first two lines are exactly correct.
To pass both challenges using a regular for loop you'd want this:
var section = document.querySelector('section');
var paragraphs = section.children;
for (let i=0; i < paragraphs.length; i++ ) { // Make sure to use semicolons and not commas here
paragraphs[i].style.color= 'blue'; // Use paragraphs, not section, since that's the collection you're intending to modify
}
Although I opted to use a for/of loop (which also passed) like this:
var section = document.querySelector('section');
var paragraphs = section.children;
for (let p of paragraphs) {
p.style.color = 'blue';
}
But either way passes and therefore both are reasonable ways to achieve the right result.
I hope that helps.
Stay safe and happy coding!
Hanwen Zhang
20,084 PointsHanwen Zhang
20,084 PointsThank you Peter