Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial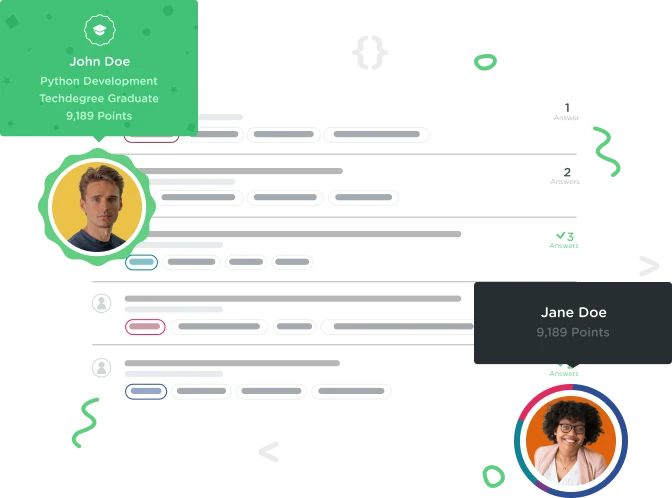
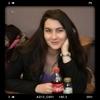
ioana morosanu
3,859 Pointswhy my "do...while loop" is not working
It gives me "Try again!". I been over it many time, but I can't see what is going wrong.
var secret = false;
do {
var secret = prompt("What is the secret password?");
if ( secret === "sesame") {
secret = true;
}
} while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers
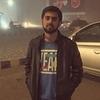
zainsra7
3,237 PointsHi ioana,
The problem is the syntax of do while loop you have written, let's look at the challenge and solve it step by step :
Here's the code they gave you and want you to convert this while loop into do-while loop:
var secret = prompt("What is the secret password?");
while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
The above code works in 3 steps:
- Ask user 1st input "What is the secret password" and store it in secret variable.
- Check while condition (secret !== "sesame") , that is if user entered sesame for the first time then don't go inside the loop and for other inputs go inside the loop. Inside loop it again ask the user "What is the secret password?" again and again until user enters "sesame" which will make While condition false and we will go to 3rd step.
- Print in document "You know the secret password, Welcome".
Now the thing is that, what if at step 1 user had entered "sesame" ? Then simply we wouldn't have gone inside the loop even a single time. But what if we wanted a way to at least execute loop body once , no matter what user entered. We just want to go inside the loop once.
So for that we have do-while loop , which lets you go inside the loop and then checks the condition of while loop
Syntax of writing do-while loop :
do {
//body of loop
}while(condition);
See above that we first go inside the loop and then while condition comes.
Let's write the final solution now :
var secret;
do{
secret = prompt("What is the secret password?");
} while ( secret !== "sesame" ) ;
document.write("You know the secret password. Welcome.");
I hope it answers your question , Happy Coding :)
- Zain

rtholen
Courses Plus Student 11,859 PointsYou are asked to replace a "while loop" with a "do while loop", but your code still has the original "while loop" at the end. On the "while" line try removing the entire block of code from the opening curly brace to the closing one and replace by a semicolon to close out the "do while" loop.
You don't need the "if" block of code because that question is already being asked in the "while" line. It will already start the loop over if they don't match and exit the loop if they do.
You are declaring the "secret" variable twice (var secret). You only need to do this once and then use it after that without the "var" keyword.
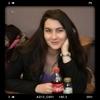
ioana morosanu
3,859 PointsPerfect! Now I got it. Thank you Zain!