Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial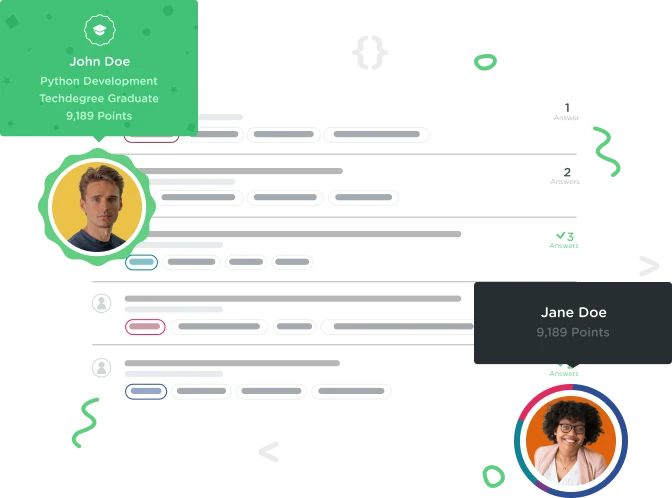
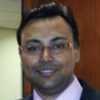
Abhijit Das
5,022 PointsWhy my event.target isn't working?
ok please help me to find the issue, my html is
<input type="text">
my css is
.bg {
background-color:#777;
outline:none;
border:solid 2px tomato;
}
and my js
const input = document.querySelector('input');
input.addEventListener('click', (e) => {
if (e.target.tagName === 'INPUT') {
input.className = "bg";
} else {
input.className = "";
}
});
once i click the input element the "bg" class name is adding, however, why it isn't removing, once i click on outside of input element? Sorry can't put the the code-pen link this time
1 Answer
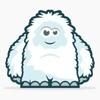
ywang04
6,762 PointsWhen you click on outside of input element, which will trigger a new event on other elements you clicked instead of input element itself. Therefore, the if else block won't be executed.
//won't be executed
if (e.target.tagName === 'INPUT') {
input.className = "bg";
} else {
input.className = "";
}
To meet your requirement with event object, you can refer to the following codes:
const input = document.querySelector('input');
input.addEventListener('mouseover', (e) => {
if (e.target.tagName === 'INPUT') {
input.className = "bg";
}
});
input.addEventListener('mouseout', (e) => {
if (e.target.tagName === 'INPUT') {
input.className = "";
}
});
or WITHOUT event object to meet your requirement :
const input = document.querySelector('input');
input.addEventListener('mouseover', () => {
input.className = "bg";
});
input.addEventListener('mouseout', () => {
input.className = "";
});