Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial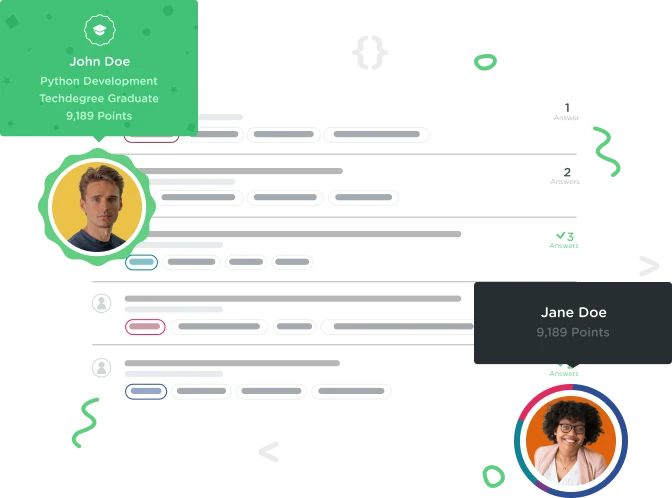
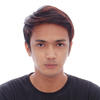
Karl Michael Figuerrez
963 PointsWhy my items in my list just keep incrementing by just pressing enter without typing any characters?
why is that?
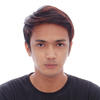
Karl Michael Figuerrez
963 Pointsnope i don't have that, i did exactly as knneth love did.

markmneimneh
14,132 Pointsdo you mind pasting the code ?
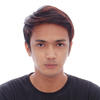
Karl Michael Figuerrez
963 Points#make a list to hold onto our items
Shopping_list = []
def show_help():
#print out instruction on how to use the app
print("What should we pick up at the store?")
print("""Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list""")
def show_list():
#print out the list
print("Here's your list: ")
for item in Shopping_list:
print(item)
print("You got {} items.".format(len(Shopping_list)))
def add_to_list(new_item):
#add new items to out list
Shopping_list.append(new_item)
print("Added {}. List now has {} items. ".format(new_item, len(Shopping_list)))
show_help()
def main():
while True:
#ask for new items
new_item = input(">")
#be able to quit the app
if new_item == 'DONE' or new_item == 'done':
break
elif new_item == 'HELP' or new_item == 'help':
show_help()
continue
elif new_item == 'SHOW' or new_item == 'show':
show_list()
continue
add_to_list(new_item)
show_list()
main()

markmneimneh
14,132 Pointshello
look at this
#be able to quit the app
if new_item == 'DONE' or new_item == 'done':
break
elif new_item == 'HELP' or new_item == 'help':
show_help()
continue
elif new_item == 'SHOW' or new_item == 'show':
show_list()
continue
add_to_list(new_item)
hint ... so what happened if your input does not meet the condition 'DONE' or new_item == 'done':
or your input does not meet the condition new_item == 'HELP' or new_item == 'help':
or your item does not meet the condition elif new_item == 'SHOW' or new_item == 'show':
...
...
...
it will
add_to_list(new_item)
so regardless of the programming language you are using ... you need to handle the case when a user enter nothing (as in blank ... or nit enter
hope this helps
2 Answers

Brandon Kelly
3,808 PointsHey there, Karl! The reason that your program adds an item to the list even though you just hit enter and didn't type anything is because Python is accepting a 'blank' as an input string. There is a method called .isalpha() that will determine whether a string is made up of alphabetic characters or not. This method returns a Boolean value(True or False). To use this method in your code you need to nest the if, elif, and else statements all within an if statement that uses the .isalpha() method.
Ex.: if shopping_list.isalpha()
if new_item == "HELP"
show_help()
else:
show_list()
else:
print("INVALID INPUT. MUST ENTER ALPHABETIC CHARACTERS ONLY")
Just an example of how that function could be used as to stop blank or numeric input. Hope this helps, I know its not quite within the scope of the lesson, but I was annoyed by my program doing the same thing and wanted to fix it.

Murali KRISHNA Devarakonda
734 PointsThe answer lies in one of the previous classes on boolean- checking for empty string. Add this to the top of your add_to_list(new_item) function.
if not (new_item):
return
markmneimneh
14,132 Pointsmarkmneimneh
14,132 Pointswithout looking at the challenge ... this means that you have something like this in the code
check you code and you should see it somewhere