Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial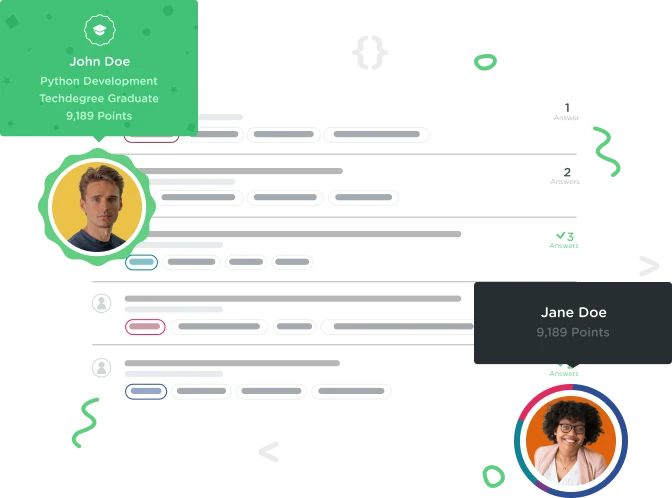

Daniel Matyka
5,719 PointsWhy my solution is not working?
Here is my code:
var html = '';
var red;
var green;
var blue;
var rgbColor;
function color() {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
return rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
}
for(var i=0; i>10; i=+1) {
html = '<div style="background-color:' + color() + '"></div>';
document.write(html);
}
Everything is working good unless i put it into loop. What i made wrong?
1 Answer

Aaron Martone
3,290 PointsHere is your code, formatted:
var html = '';
var red;
var green;
var blue;
var rgbColor;
function color() {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
return rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
}
for (var i=0; i>10; i=+1) {
html = '<div style="background-color:' + color() + '"></div>';
console.log(html);
}
Some of the problems I note is: (1) Your return
value for your function should not assign that value to rgbColor
, but simply just return it, like return 'rgb(' + red + ',' + green + ',' + blue + ')';
. Secondly, your loop has problems. The condition states to only run the loop if i>10
. i
will never be > 10 because you initialized it to 0
, so the loop never runs. Also, where you increment it, i=+1
is an invalid statement. Use i++
. So the fixed version should look like:
var html = '';
var red;
var green;
var blue;
var rgbColor;
function color() {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
return 'rgb(' + red + ',' + green + ',' + blue + ')';
}
for (var i=0; i<10; i++) {
html = '<div style="background-color:' + color() + '"></div>';
document.write(html);
}
rydavim
18,814 Pointsrydavim
18,814 PointsI've changed your comment to an answer so that it may be voted on or marked as best. Thanks for participating in the community!
Aaron Martone
3,290 PointsAaron Martone
3,290 PointsThanks rydavim. I misinterpreted the UI, thinking that comments could be MARKED as answers by the OP once they were deemed correct. I will try using the ANSWERS interface when I think I'm providing an answer rather than the COMMENT one. Thanks for the clarification.