Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial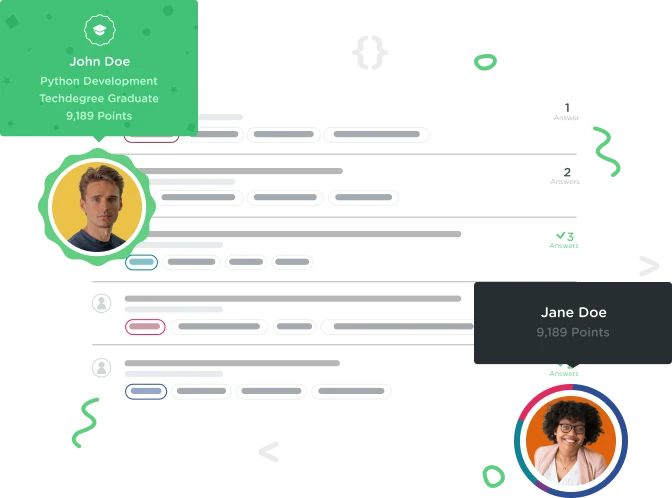

lassek
8,209 PointsWhy not bind in the constructor?
In this video, the preferred solution suggested to binding is to use arrow methods...but wouldn't it be a cleaner solution to just bind the method in a constructor? It just feels wrong having to divert from the class method paradigm to solve this, when there already is an easy solution...or is the constructor solution bad in some way?
I also feel that this video rushed through this, immediately changing to testing the code after writing it. Just a bit of delay to allow pausing in time to see the actual code written would not hurt.
EDIT: I saw now that constructor binding is mentioned in the teachers notes. But still: wouldn't this be the most elegant solution?
2 Answers
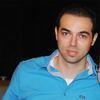
Ezra Siton
12,644 PointsFirst, this is not why not
- this is one recommended approach to solve the idea of binding (You can use a constructor - you find examples under React docs).
"THE PROBLEM"
You should not call setState()
in the constructor()
= extra lines ==> Less readable + Harder to maintenance.
https://reactjs.org/docs/react-component.html#constructor
Bind in constructor
constructor() {
super();
this.state = { score: 0 };
this.incrementScore = this.incrementScore.bind(this);
}
incrementScore(){
this.setState({
score: this.state.score + 1
});
}
Bind in Render
The option below is more readable than this option (Imagine you work with 50 methods, not 1 - and you should remember for each one to add extra lines inside the constructor).
state = {
score: 0
};
incrementScore = () => {
this.setState({
score: this.state.score + 1
});
}
On the button click event:
<button className="counter-action increment" onClick={this.incrementScore.bind(this)}> + </button>
This article sums well this issue:

fairest
Full Stack JavaScript Techdegree Graduate 19,303 PointsPlease note, various style guide warn not to use the arrow function. Airbnb classifies is as very bad practice: Do not use arrow functions in class fields, because it makes them challenging to test and debug, and can negatively impact performance, and because conceptually, class fields are for data, not logic.
lassek
8,209 Pointslassek
8,209 PointsThanks Ezra for your excellent reply. It was also good to read that article with the decision tree. I guess to me it felt intuitively wrong to not use the class/method paradigm in order to fix this (pun intended:-). But if there are more than a few methods then ofc it gets messy.