Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial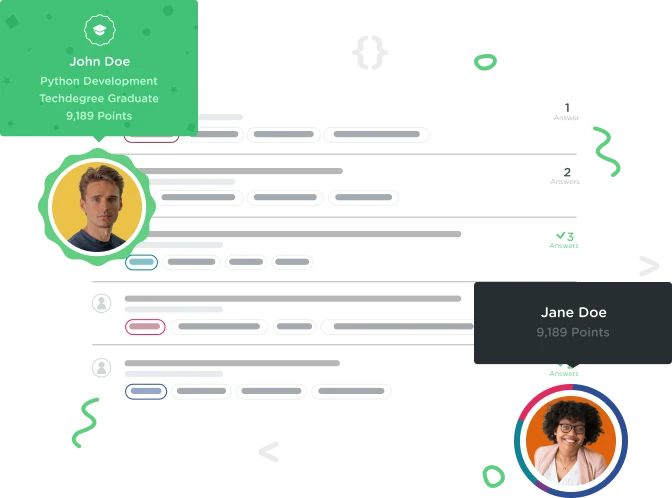

Devanshu Kaushik
18,946 PointsWhy not use a Review object directly with gson instead of a Map while writing tests?
We could simply go for:
//0 used as courseId as a default value.
Review review = new Review(courseId: 0, rating: 4, comment: "test review");
.
.
.
ApiResponse response = client.request("POST", "/courses/"+course.getId()+"/reviews", gson.toJson(review));
Any reasons we shouldn't do so?
1 Answer
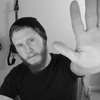
Jeremiah Shore
31,168 PointsTLDR: it helps to clarify your test code.
We've designed the POST request of the API so that it is expecting two attributes/values, and it also pulls the course_id from the url params. Using gson.toJson(review)
includes all fields from that object.
@Test
public void exampleReviewJsonOutputMapVsGson() {
//Map
Map<String, Object> values = new HashMap<>();
values.put("rating", 3);
values.put("comment", "it was okay");
System.out.println(gson.toJson(values));
//Gson with Review.class
Review review = new Review(course.getId(), 3, "it was okay");
System.out.println(gson.toJson(review));
}
/* console output:
{"rating":3,"comment":"it was okay"}
{"id":0,"course_id":1,"rating":3,"comment":"it was okay"}
*/
When using the API, and specifically when POSTing reviews, there actually doesn't seem to be any harm in using Gson how you've described it.
course_id
is overwritten before persisting because we're manually setting it with .setCourseId()
in the post route after we deserialize from json. The id
is also ignored, because that's a value generated by Sql2o when it persists to the database, and it's not even in our ReviewDao implementation. If it was, I believe it would cause a SQL error if we tried to set it manually.
Very important to consider is how clients will be submitting data to your API in their requests. Using a map is more representational of how the data will be submitted—attriutes supplied in key/value pairs. Hopefully, if this was a production system, you'd have developer facing documentation describing what types of requests can be made (POST/GET), which URLs they can be submitted to (our defined routes), the necessary headers (Content-Type of "application/json"), and which key/value attributes they need to include in their json requests.