Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial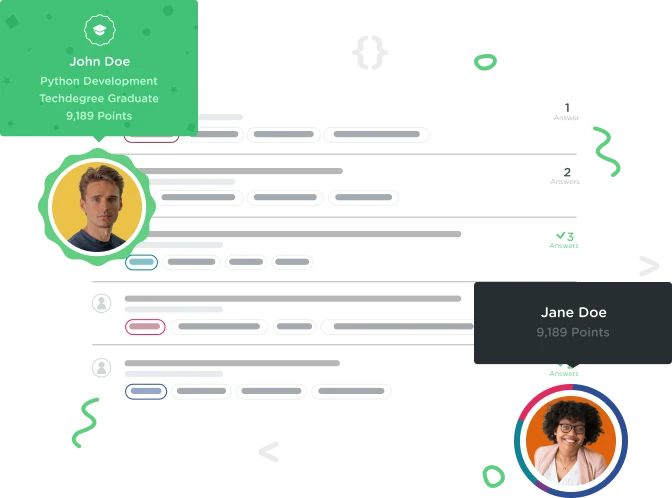

William Elias
9,472 Pointswhy not use an "and" in the while loop instead of the "if"?
import sys
password = input("please enter the super secret password: ") attempt_count = 1 while (password != 'opensesame'): if attempt_count > 3: sys.exit("Too many invalid password attempts") password = input("invalid password, try again: ") attempt_count += 1 print("Welcome to secret town")
vs
password = input("please enter the super secret password: ") attempt_count = 1 while (password != 'opensesame') and (attempt_count < 3): password = input("invalid password, try again: ") attempt_count += 1 print("Welcome to secret town")
2 Answers
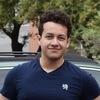
James Estrada
Full Stack JavaScript Techdegree Student 25,867 PointsThe problem for having
while ((password != 'opensesame') and (attempt_count < 3)):
is that once the attempt_count no longer holds true, the loop will exit, and it will still print "Welcome to secret town"; which it should be printed only if the password was correct.

Philipp Räse
Courses Plus Student 10,298 PointsHello William,
first of all I felt free to convert your could a bit nicer to read
Your Code:
import sys
password = input("please enter the super secret password: ")
attempt_count = 1
while (password != 'opensesame'):
if attempt_count > 3:
sys.exit("Too many invalid password attempts")
password = input("invalid password, try again: ")
attempt_count += 1 print("Welcome to secret town")
vs
import sys
password = input("please enter the super secret password: ")
attempt_count = 1
while (password != 'opensesame') and (attempt_count < 3):
password = input("invalid password, try again: ")
attempt_count += 1
print("Welcome to secret town")
The mistake:
It would work with an and
statement inside the while condition. But unfortunately you forgot an additional pair of brackets to surround your logic while-condition.
The solution:
The following code contains the missing brackets.
import sys
password = input("please enter the super secret password: ")
attempt_count = 1
while ((password != 'opensesame') and (attempt_count < 3)):
password = input("invalid password, try again: ")
attempt_count += 1
print("Welcome to secret town")
I hope my answer helped you out.
Sincerly,
Phil

William Elias
9,472 PointsThanks Phil!
I know there is more than one way to accomplish a task, but is there a more correct way here? Or will a later lesson make this question irelavent?

Philipp Räse
Courses Plus Student 10,298 PointsYou're welcome William,
I think that I cannot answer your question that easy. It is much about personal preferences.
Craig Dennis mentioned in another curse the KISS-principle. Keep-It-Stupidly-Simple
But if you're writing comments that will describe your code, you and others will have it much easier understanding even complex lines of code.
The best code-structure is quite subjective and depends much on the relating project.
I would say, you better follow up the next lessons and develop your own view. I'm sure you'll do it great! :)