Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial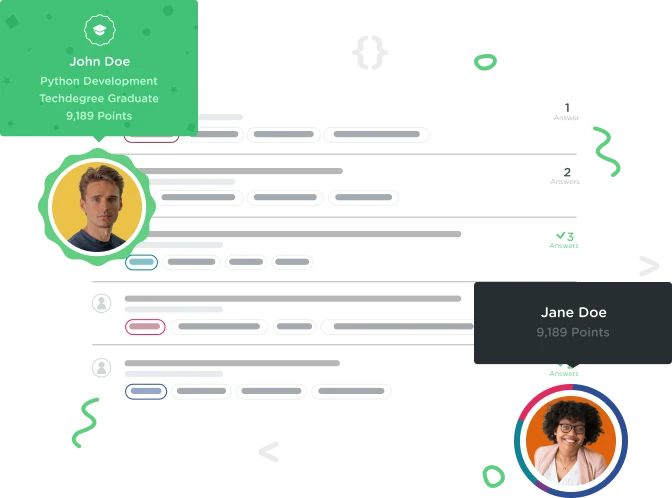
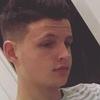
Nick Evershed
570 PointsWhy not use If instead of using Elif
^^^
3 Answers

Sebastian Rother
6,715 PointsIt simply prevents your other if-statements from being checked against.
Example: Let's assume you have a variable containing an age of 18 and you are checking if the person is an adult or still a child.
Using only if statements...
age = 18
if age < 21:
print("You are a child")
if age >= 21:
print("You are an adult")
else:
print("The age must be a positive integer!")
...will make the person an adult and a child at the same time.
But elif...
if age < 21:
print("You are a child")
elif age >= 21:
print("You are an adult")
else:
print("The age must be a positive integer!")
...will define the person only as a child.
Bonus: To achieve the same result just with if and else, it would look like this:
if age < 21:
print("You are a child")
else:
if age >= 21:
print("You are an adult")
else:
print("The age must be a positive integer!")
The more checks you are implementing, the more levels of indentation you will get, wich makes it not that easy to handle. Sometimes referred to as 'Pyramid of Doom'.

Colton Ehrman
255 PointsNot sure what you are asking without any context, but elif
allows you to have multiple conditions in a single waterfall like structure that flows down each conditional and executes the first one that is true.
If you only have an if
statement, more complex conditions would not be usable, or extremely hard to implement.

amyyxt
2,227 PointsAlso, because it prevents the following elif-statements from being checked against, the computer technically runs the script faster since it doesn't have to check any elif statements that come after the first true one. This will matter when you write longer code with more if statements.
BTW, Richard Li's correction about Sebastian Rother's example was correct.
Richard Li
9,751 PointsRichard Li
9,751 PointsI think you mean that age variable in the first double if condition will be checked twice by child and adult and based on the syntax it will return a child and The age must be a positive integer at the same time.