Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial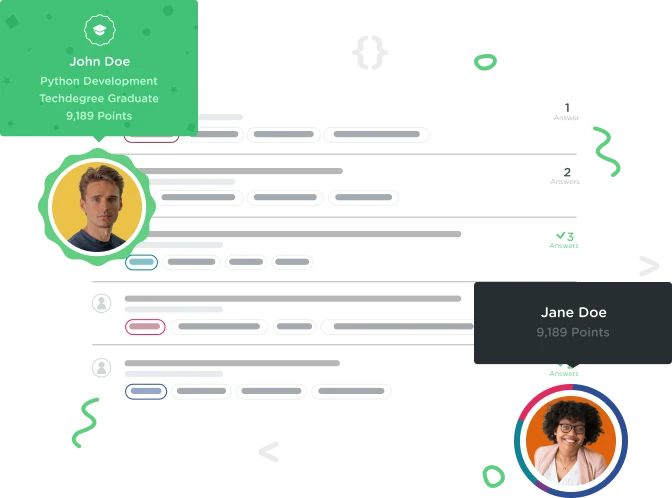

Antonio Ascue Avalos
3,023 PointsWhy not use let instead of const for arrays and objects?
The question is in the title thanks.
2 Answers

Wiktor Bednarz
18,647 PointsHey Antonio,
your question is quite broad, but yet it assumes a false statement. Arrays and object, as well as any data type can be assigned using let and const declarations. The declarations you use just give your variable some restrictions that it's got to follow.
The differences between const and let are however miniscule and more conceptual than pragmatic. The only real difference between them is that const variables are partially immutable, and let variables aren't. What that means is that when you declare a variable in your code with const, then you can't reassign it to a different value.
let x = 'foo';
const y = 'foo';
x = 'bar'; // this doesn't throw you an error
y = 'bar'; // this throws you a TypeError
Why is const partially immutable then? Because it can still be manipulated with operations performed on it
const x = 0;
x+=1 // this throws you a TypeError
/*******************************************************************************/
const foo = {};
foo = { bar: x }; // TypeError again
foo.bar=x // this however doesn't throw you an error and when you log it:
console.log(foo);
// { bar: 0 }
So that's why const variables aren't absolutely immutable.
Following the trope of your question now, it's broadly considered a good practice to declare data such as arrays and objects as const only because the fact that you mostly want it to be assigned only once and perform any changes to them with appropriate methods.
If you want to read more about the topic, please refer to MDN let and const documentation, or have a look at this article.
Hope this helps,
Wiktor Bednarz
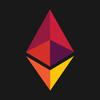
Richard Verbraak
7,739 PointsConst is simply used when you don't want the IDENTIFIER changed.
Copied from MDN:
Constants are block-scoped, much like variables defined using the let statement. The value of a constant cannot change through reassignment, and it can't be redeclared.
Let is used when you want it's value to be changed, like a for-loop counter variable but it's local scope. Meaning, that variable will only be used in the codeblock it's defined in.