Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial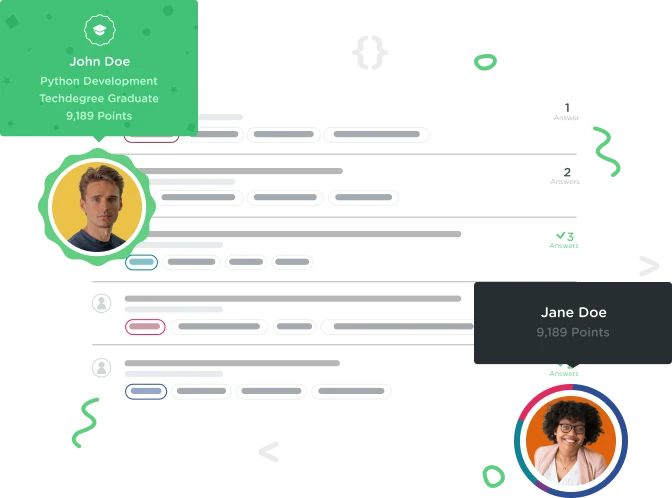
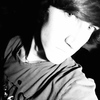
Christopher St. George
2,606 PointsWhy not use playlistDetailController.loadView()?
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
if segue.identifier == "showPlaylistDetail" {
if let playlistDetailController = segue.destinationViewController as? PlaylistDetailViewController {
playlistDetailController.loadView()
playlistDetailController.buttonPressLabel.text = "You pressed the button!"
}
}
}
2 Answers

Chris Shaw
26,676 PointsHi Christopher,
The default segues built into Xcode are already designed to handle this for us, anytime the prepareForSegue
method gets called on the parent view a new instance of the class
is created, once the prepareForSegue
has finished executing, meaning once our custom code is done it then loads the view automatically for us.
Hope that helps.
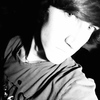
Christopher St. George
2,606 PointsI understand that the view is loaded automatically for us, but I guess what I would like to know is if what I did is bad practice or not.
In the Modifying the UI video, Pasan mentions that we cannot modify the UILabel object within the prepareForSegue method because the view is not fully loaded yet. By calling the loadView method, it would seem that this is no longer an issue.
class PlaylistMasterViewController: UIViewController {
@IBOutlet weak var aButton: UIButton!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
aButton.setTitle("Press me!", forState: .Normal)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
if segue.identifier == "showPlaylistDetail" {
// NOTE: In the video we did not use an 'if let' statement, but simply forced the downcast
// I just prefer not forcing it.
if let playlistDetailController = segue.destinationViewController as? PlaylistDetailViewController {
playlistDetailController.loadView()
playlistDetailController.buttonPressLabel.text = "You pressed the button!"
}
}
}
}
class PlaylistDetailViewController: UIViewController {
@IBOutlet weak var buttonPressLabel: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}

Noel Deles
8,215 PointsI option clicked the method within XCode and here is an excerpt of what popped up:
"You should never call this method directly. The view controller calls this method when its view property is requested but is currently nil. This method loads or creates a view and assigns it to the view property. If the view controller has an associated nib file, this method loads the view from the nib file. A view controller has an associated nib file if the nibName property returns a non-nil value, which occurs if the view controller was instantiated from a storyboard, if you explicitly assigned it a nib file using the initWithNibName:bundle: method, or if iOS finds a nib file in the app bundle with a name based on the view controller's class name. If the view controller does not have an associated nib file, this method creates a plain UIView object instead.
If you use Interface Builder to create your views and initialize the view controller, you must not override this method."
In bold are key points I wanted to highlight. So I'm guessing that yes, it is bad practice. HTH.
Jun Da Ma
2,266 PointsJun Da Ma
2,266 PointsHi I am wondering the same thing as well, whether this is a bad practice or not.
Would anyone be able to advice?