Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial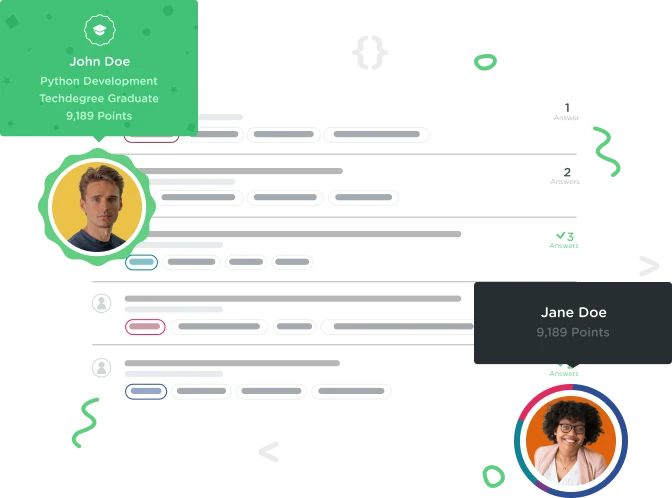

Jonathan Fernandez
1,423 PointsWhy not use prototype for Song.isPlaying?
I understand that this.title, this.artist, and this.duration need to stay in the constructor function as they are arguments when we create a new instance of song. However I have a question about this.isPlaying:
Since using a prototype helps save on Ram/efficiency, why not use it on this.isPlaying to Song.prototype.isPlaying rather than keeping it in the constructor function to run each time a new instance of song is made?
function Song(title, artist, duration) {
this.title = title;
this.artist = artist;
this.duration = duration;
// this.isPlaying = false; //make a prototype?
}
Song.prototype.isPlaying = false; // <--
3 Answers
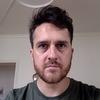
Raphaël Seguin
Full Stack JavaScript Techdegree Graduate 29,228 PointsHi Johnatan, I think it would be wrong because you want to know if a particular instance of Song is playing so this variable should be created for every instance. You can define methods on the prototype because the function would be the same for every Song but every song has different variables. Every song has different properties but they have methods in common. Does it make sense to you ? Cheers,
Raphaël

Sean Smith
13,797 PointsI think we want to reserve the prototype for functions, not Boolean values. isPlaying is a property of Song

Cameron Chong
4,298 PointsJonathan Fernandez, javascript isn't a compiled language like C#. You aren't wasting compiler time because there isn't one. The reason for putting methods on the prototype is to save memory. If you want to do some googling, think of them as variables by reference, not by value.
Jonathan Fernandez
1,423 PointsJonathan Fernandez
1,423 PointsHi Raphael, thanks for the answer. I'm still a bit confused because, I had wrote my code with the isPlaying as a prototype and it still works. From my understanding with MDN the new already creates the property for the Song Object every time we assign a new instance to a variable and I'm able to access it and change it locally to individual vars new Song was made under.
MDN: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/new
I'm still trying to grasp prototypes but I believe we are excluding functions because we don't want the functions' large code to use up compiler time every time we create a new object so we just refer to it's name. Does using prototype for properties help with efficiency by not having to run through each of them each time we create a new object?
E.g. function Object() { this.property (x 500000000 times) = value; } -- vs -- Object.prototype.property (x 5000000 times) = value
Will var object = new Object save on having to execute the 5000000 lines of this.property if I create it through prototype? Are prototyping properties efficiently done through a prototype reference or are all prototype.property declarations also being run through the compiler? (Hope this make sense, otherwise I could seriously be confusing myself haha)
gennady
14,145 Pointsgennady
14,145 PointsGreat questions! I'm waiting for answers either! Thanks! =)