Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial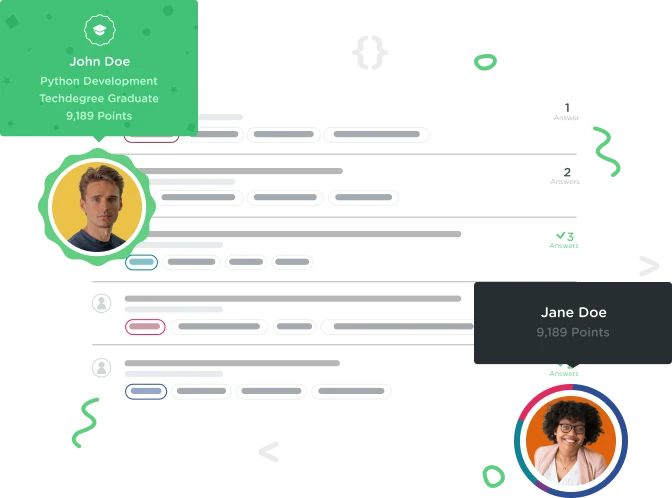
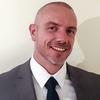
Jason Welsh
Treehouse Project ReviewerWhy not use string.startsWith() instead?
For the second challenge, instead of using the charAt() method, why not use the cleaner and more readable ES6 'startsWith()' method?
ex:
const names = ["Selma", "Ted", "Mike", "Sam", "Sharon", "Marvin"];
let startsWithS = [];
names.forEach(name => {
if (name.startsWith("S")) {
startsWithS.push(name);
}
});
console.log(startsWithS);
4 Answers
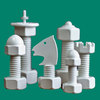
Steven Parker
231,269 PointsYour suggestion does make a good alternative solution.
Out of curiosity, I bench-marked both approaches and found the original "charAt" solution to be about 30% more efficient. But I doubt it was selected for execution speed. It was probably just considered to be a more commonly used string method (and that may be just because it has been around longer).
As Joseph pointed out, there are often multiple ways to solve any specific problem. One of "Parker's Rules of Programming" is "the more complex the task, the more ways there will be to arrive at a solution".

Joseph Wasden
20,407 PointsYou'll hear the instructor say a few key things during the video. Before solving, he states, "here's how I solved this," and, before using the charAt(), he says, (rough paraphrasing ahead) "I need to check the first letter of each word to know if I should add it to my sNames array. You could do this a couple ways. I used the charAt() method."
In other words, there are many ways to solve a problem, and many would work here. If you really needed it, you could research which method is most optimized for performance, but given this exercise, the difference is probably negligible.
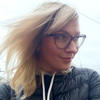
Olga Ioffe
Front End Web Development Techdegree Graduate 19,955 PointsI personally used startsWith() for my solution. I think it's semantically more readable what my code is doing here.
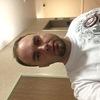
Kodie Ivie
Full Stack JavaScript Techdegree Graduate 21,250 Pointsyou know what they say: "ask 20 programmers to solve the same problem and you'll get 20 different ways of doing it". I myself used the slice method.. but i was surprised we both used sNames lol..
let sNames = [];
names.forEach(name => { if(name.slice(0, 1) === 'S'){ sNames.push(name); } });
nfs
35,526 Pointsnfs
35,526 PointsI seriously googled: Parker's Rules of Programming;
Steven Parker
231,269 PointsSteven Parker
231,269 Points