Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial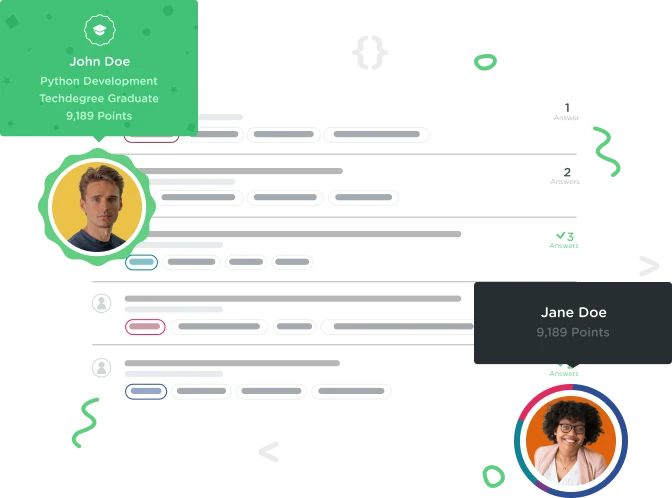
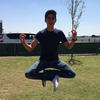
Andrés Leal
9,563 PointsWhy Pasan used a Struct for Point?
Why does he used a struct for Point instead of using a class named Point, would it be the same to use a class?
I have changed the Point type to class and it works the same.
Thanks in advance
1 Answer
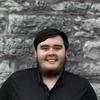
Michael Hulet
47,912 PointsNo, it wouldn't be the same. struct
s are value types, but class
es are reference types. For example:
struct ValuePoint{
var x: Int
var y: Int
}
let firstValue = ValuePoint(x: 0, y: 1)
var secondValue = firstValue
secondValue.x = 3
print(firstValue.x) // Prints 0
print(secondValue.x) // Prints 3
class ReferencePoint{
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
let firstReference = ReferencePoint(x: 0, y: 1)
let secondReference = firstReference
secondReference.x = 3
print(firstReference.x) // Prints 3
print(secondReference.x) // Prints 3
A few things to note there:
- The
class
makes you write your own initializer, butstruct
s will synthesize it for you - Changing the
x
property ofsecondReference
will also change that property offirstReference
because the settingsecondReference
to befirstReference
will copy over the reference to the object infirstReference
, but bothfirstReference
andsecondReference
will both be pointing to the same underlying object. However,firstValue
andsecondValue
contain the objects themselves, and so when you setsecondValue
to befirstValue
, a whole new object copied to have the same property values of the one infirstValue
will be created and given tosecondValue
. SincefirstValue
andsecondValue
contain different objects, updating one will not update the other -
secondValue
needs to be a variable, because it directly contains the object itself and its properties, so to make the properties of the object mutable,secondValue
itself needs to be mutable.firstValue
can be a constant because we're not changing it at all. However, bothfirstReference
andsecondReference
can be constants, because they only contain references that they never change. The underlying object itself that they both point to is mutable, so we can still change itsx
property, even though we're referencing it through a constant
In general, class
es are meant to actually do things, but struct
s are meant to just contain some data. Point
is an awesome candidate for a struct
because it's just meant to be a structured wrapper around some data, and it's not really meant to perform much/any functionality on its own