Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial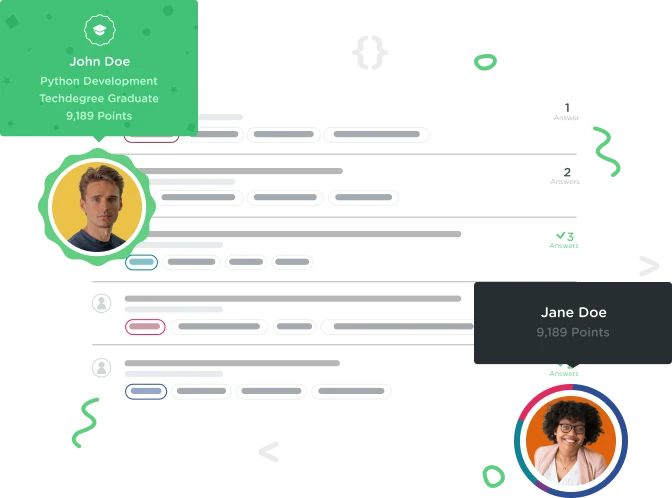
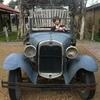
Brendan Moran
14,052 PointsWhy pass a destructured object to the constructor?
Does the class constructor need to receive a data object? Can it not receive regular parameters? (Going to check the MDN docs... Ok, yes, you CAN pass any value.)
So, why do this...
class Student {
constructor({name, age, interestLevel = 5} = {}) {
this.name = name;
this.age = age;
this.interestLevel = interestLevel;
this.grades = new Map()
}
}
let sarah = new Student({ name: 'Sarah', age: 22, interestLevel: 9 });
When you could just do this...
class Student {
constructor(name, age, interestLevel = 5) {
this.name = name;
this.age = age;
this.interestLevel = interestLevel;
this.grades = new Map()
}
}
let sarah = new Student('Sarah', 22, 9);
3 Answers
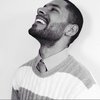
Michael Liendo
15,326 PointsYou can absolutely do it your way. The main (only?) reason not to, would be to have self commenting code. Imagine your example but in a larger project setting where the class was defined in it's own file somewhere and you came across
let sarah = new Student('Sarah', 22, 9);
You wouldn't know what 22 or 9 referenced. You have to write a comment for yourself or someone else, not just there, but every time someone create a new student. By using an object, we know that 22 is age and not years-until-student-loans-are-paid-off.

Lars Reimann
11,816 PointsIn addition to self-documentation of function calls, destructuring is useful if multiple parameters are optional. In the example from earlier where you created a string containing a greeting and a name both were optional:
function greet(greeting = 'Hey', name = 'Brendan') {
return `${greeting}, ${name}`;
}
If you want to change only the name, you need to pass in an explicit undefined: greet(undefined, 'Lars')
. If you would swap the parameters, you would run into the same problem if you just want to change the greeting. This is because the mapping from actual to formal parameters depends only on the position.
In Python you could use named parameters and, using destructuring, you can emulate this feature:
function greet({greeting = 'Hey', name = 'Brendan'} = {}) {
return `${greeting}, ${name}`;
}
Now, you can change just one of the default values when calling the function: greet({name: 'Lars'})
. And more often than not, you will benefit from property value shorthands, because you already have a variable with the correct identifier. (here name). Then the call become even shorter: greet({name})
.
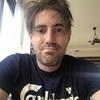
andreavitiani
13,313 Pointsthis is the question/answer I was looking for.
Is there any other important reason one should use this syntax?
Brendan Moran
14,052 PointsBrendan Moran
14,052 PointsMakes sense, Michael! That's what I was looking for.