Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial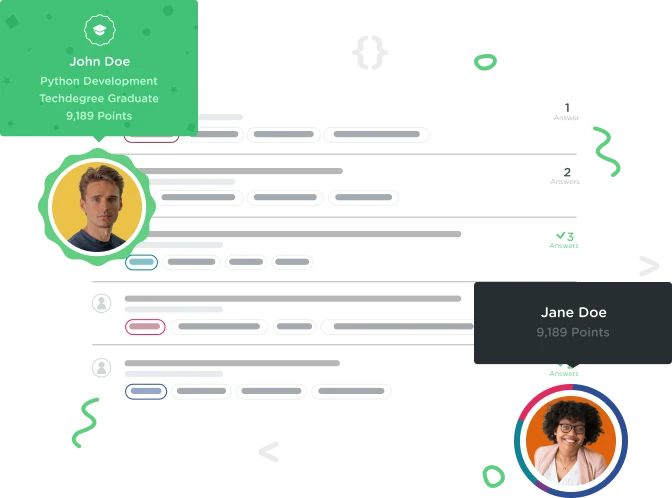
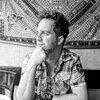
jlampstack
23,932 PointsWhy pass {} = {} to constuctor?
What is the importance of passing the second object?
Why write....
class Student {
constructor( {name, city, interestLevel = 5} = {} ) {
this.name = name;
this.city = city;
this.interestLevel = interestLevel;
}
}
let john = new Student({ name: 'John', city: 'Portland', interestLevel: 5});
console.log(john);
When we can write....
class Student {
constructor( {name, city, interestLevel = 5} ) { // This is the only line that is different
this.name = name;
this.city = city;
this.interestLevel = interestLevel;
}
}
let john = new Student({ name: 'John', city: 'Portland', interestLevel: 5});
console.log(john);
3 Answers
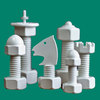
Steven Parker
231,275 PointsThat first set of braces define the kind of object that the constructor will take. If you have that by itself, then every instantiation must supply an object.
The "= {}
" part afterwards establishes a default, so if the constructor is called without an argument, an empty object will be supplied. This makes the argument optional instead of mandatory when you create an instance.
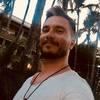
Peter Retvari
Full Stack JavaScript Techdegree Student 8,392 PointsHi folks, how about this one? I don't understand why we use curly braces:
class Student {
constructor(name, age, interestLevel = 5) {
this.name = name;
this.age = age;
this.interestLevel = interestLevel;
}
}
let joey = new Student('Joey', 25);
let chandler = new Student('Chandler', 26);
console.log(joey);
console.log(chandler);
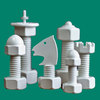
Steven Parker
231,275 PointsYou'd have the same issue as Jay then, you could only create an instance if you supply a name and age. The purpose of passing the arguments through an object, and having a default object, is that it allows you to create an instance with no arguments if you want:
let someone = new Student();
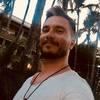
Peter Retvari
Full Stack JavaScript Techdegree Student 8,392 PointsWow, thanks, Steven. I got it now.